如何在单链表中实现元素的插入和删除操作?
时间: 2024-10-11 09:17:58 浏览: 47
在单链表中实现元素的插入和删除操作通常涉及以下几个步骤:
1. **元素插入**:
- **在链表头部插入**: 如果要插入在列表的开始位置,新节点会作为头节点,然后将原头节点的指针指向新节点,新节点的next指针指向原来的第一项。
- **在链表尾部插入**: 遍历整个链表找到最后一个节点,将其next指针指向新的节点,新节点的next设为null。
- **在指定位置插入**: 首先找到插入位置前的节点,然后更新这个节点的next指针指向新节点,新节点的next指针指向原目标位置的下一个节点。
2. **元素删除**:
- **删除首节点**: 如果要删除的是第一个节点(头节点),只需将链表的头指针指向第二个节点即可,如果头节点就是我们要删除的,则需设置一个新的头节点。
- **删除其他节点**: 遍历链表找到待删除节点的前一个节点,然后将其next指针跳过待删除节点,使其直接连接到下一个节点。
记得在操作过程中处理特殊情况,比如空链表、要删除的节点为空等。以下是伪代码示例:
```python
# 定义链表节点
class Node:
def __init__(self, data=None, next=None):
self.data = data
self.next = next
# 插入操作
def insert_at_head(head, new_data):
new_node = Node(new_data)
new_node.next = head
return new_node
def insert_at_position(head, pos, new_data):
if pos == 0:
return insert_at_head(head, new_data)
current = head
for _ in range(pos - 1):
if not current.next:
break
current = current.next
if not current.next:
print("Position out of bounds")
else:
new_node = Node(new_data)
new_node.next = current.next
current.next = new_node
# 删除操作
def delete_node(head, key):
if head and head.data == key:
head = head.next
return head
current = head
while current.next and current.next.data != key:
current = current.next
if current.next is None:
print("Node not found")
else:
current.next = current.next.next
```
阅读全文
相关推荐
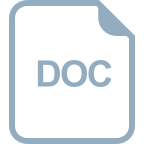
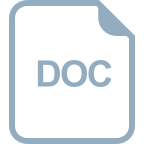
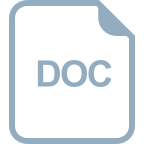



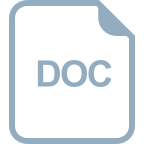
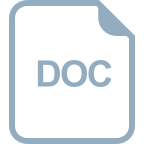
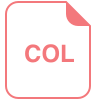









