编程实现如下功能: (1)根据输入的一系列整数,以0标志结束,用头插法建立单链表,并输出单链表中各元素值,观察输入的内容与输出的内容是否一致, (2)在单链表的第i个元素之前插入1个值为x的元素,并输出插入后的单链表中各元素值。 (3)删除单链表中第i个元素,并输出删除后的单链表中各元素值。 用c++实现
时间: 2024-02-20 17:02:12 浏览: 111
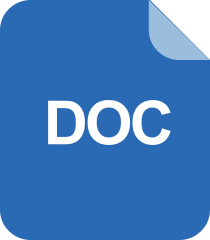
C++实现动态链表的建立、输出、插入和删除功能。

以下是用C++实现的代码:
```c++
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* createList() {
int num;
ListNode* head = new ListNode(0);
ListNode* p = head;
cout << "请输入一系列整数(以0结束):" << endl;
cin >> num;
while (num != 0) {
ListNode* node = new ListNode(num);
p->next = node;
p = p->next;
cin >> num;
}
return head->next;
}
void printList(ListNode* head) {
cout << "单链表中各元素值为:" << endl;
ListNode* p = head;
while (p) {
cout << p->val << " ";
p = p->next;
}
cout << endl;
}
ListNode* insert(ListNode* head, int i, int x) {
ListNode* p = head;
int count = 0;
while (p && count < i - 1) {
p = p->next;
count++;
}
if (p) {
ListNode* node = new ListNode(x);
node->next = p->next;
p->next = node;
}
return head;
}
ListNode* remove(ListNode* head, int i) {
ListNode* p = head;
int count = 0;
while (p && count < i - 1) {
p = p->next;
count++;
}
if (p && p->next) {
ListNode* temp = p->next;
p->next = temp->next;
delete temp;
}
return head;
}
int main() {
ListNode* head = createList();
printList(head);
int i, x;
cout << "请输入要插入的位置和值:" << endl;
cin >> i >> x;
head = insert(head, i, x);
printList(head);
cout << "请输入要删除的位置:" << endl;
cin >> i;
head = remove(head, i);
printList(head);
return 0;
}
```
其中,createList()函数用于创建单链表,printList()函数用于输出单链表中的元素值,insert()函数用于在单链表的第i个元素之前插入一个值为x的元素,remove()函数用于删除单链表中的第i个元素。
阅读全文
相关推荐


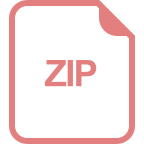
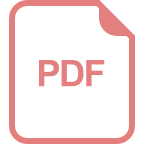
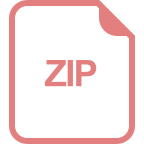
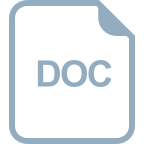
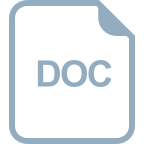
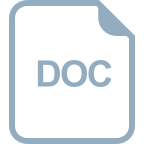
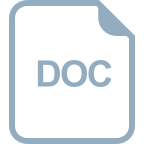
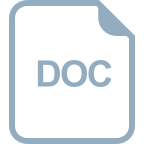
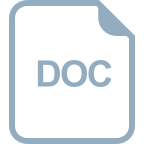
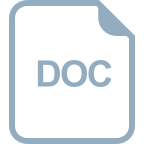
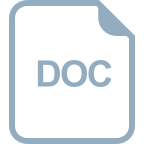
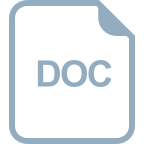
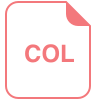
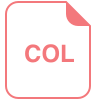