java实现音频响度归一化
时间: 2024-09-24 07:27:54 浏览: 54
在Java中实现音频文件的响度归一化,通常涉及到处理音频流并调整其幅度值,使其在整个音轨上保持一致的响度。这个过程通常包含以下几个步骤:
1. **加载音频文件**:使用Java的AudioInputStream或类似库(如JAVE、JFugue等)读取音频数据。
2. **获取采样率和通道数**:了解音频的采样频率和声道数,这对于后续处理非常重要。
3. **计算平均响度**:遍历音频数据,对每个样本点取对数(因为人耳对响度的变化敏感于音频强度的对数),然后求平均,得到当前的平均响度。
4. **标准化**:对于每个样本,减去平均响度,并乘以一个固定的阈值(例如,可以设置为0分贝),使得结果音频的平均响度达到预设的标准。
5. **保存或播放归一化的音频**:将处理后的音频数据写回一个新的AudioInputStream,或者通过AudioSystem工具播放。
以下是一个简单的示例代码片段,但这只是一个简化的版本,实际应用中需要处理线程安全和错误处理等问题:
```java
import javax.sound.sampled.AudioInputStream;
import javax.sound.sampled.AudioSystem;
public class AudioNormalization {
private static final double MAX_DB_GAIN = 0; // Set target maximum dB gain
public static void normalize(AudioInputStream audio) throws Exception {
int sampleSizeInBytes = audio.getFormat().getSampleSizeInBits() / 8;
byte[] buffer = new byte[sampleSizeInBytes];
// Get the total number of frames and their average db level
int frameCount = (int) audio.framesToMillisecond(audio.getFrameLength());
float avgDb = calculateAverageDb(audio);
// Normalize each frame to the desired max dB
while (audio.read(buffer, 0, buffer.length) != -1) {
for (byte b : buffer) {
double amplitude = Float.intBitsToFloat(BitConverter.getInt(b)) * 0.0078125f; // Convert to float
double normalizedAmplitude = Math.max(0, amplitude - avgDb) * AudioUtil.dbToLinear(MAX_DB_GAIN);
float normByte = Float.floatToIntBits(normalizedAmplitude);
buffer[BitConverter.byteOffset(normByte, 0)] = normByte;
}
// Write back the normalized audio
audio.write(buffer, 0, buffer.length);
}
audio.close();
}
private static float calculateAverageDb(AudioInputStream audio) throws Exception {
// ... implement a method to calculate average db over the stream
}
}
```
阅读全文
相关推荐
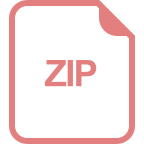
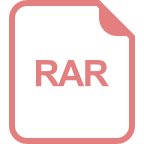
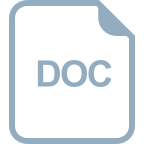
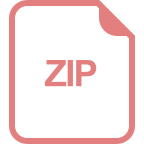
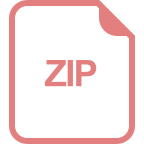
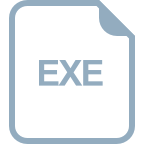
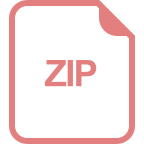
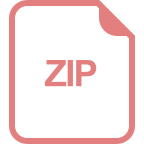
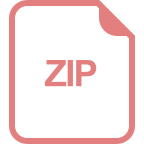
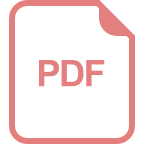
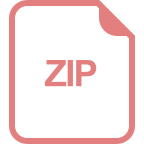
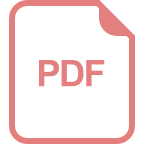
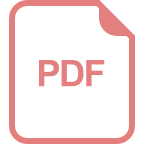
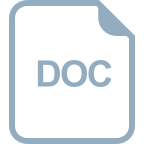
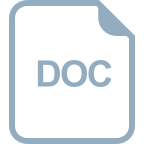
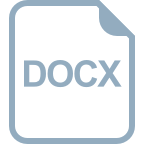
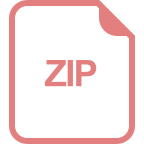