用python语言编写一个程序,使用logistic回归实现梯度下降算法,决定迭代次数,计算每次迭代并绘制结果。数据集通过txt文件输入且x和y的数据集彼此独立。使用numpy和matplotlib
时间: 2024-12-20 14:30:35 浏览: 15
在Python中,我们可以使用`numpy`库来处理数值计算,包括梯度下降和数据处理,而`matplotlib`用于可视化学习过程。下面是一个简单的示例,展示如何使用Logistic回归和梯度下降来拟合数据,并绘制出每次迭代的学习曲线:
```python
import numpy as np
import matplotlib.pyplot as plt
# 加载数据,假设数据集存储在名为data.txt的文本文件中
def load_data(filename):
with open(filename, 'r') as f:
x = [float(line.split()[0]) for line in f]
y = [int(line.split()[1]) for line in f] # 将标签转为二进制形式
return np.array(x), np.array(y)
# Logistic Regression sigmoid 函数
def sigmoid(z):
return 1 / (1 + np.exp(-z))
# 梯度下降函数
def gradient_descent(X, y, weights, learning_rate, num_iterations):
m, n = X.shape
cost_history = []
for i in range(num_iterations):
z = np.dot(X, weights) # 计算线性组合
h = sigmoid(z)
error = y - h # 错误向量
weight_derivative = -(1/m) * np.dot(X.T, error)
bias_derivative = -(1/m) * np.sum(error)
weights -= learning_rate * weight_derivative
bias -= learning_rate * bias_derivative
cost_history.append(cost_function(X, y, weights, bias)) # 记录成本函数值
return weights, bias, cost_history
# 计算代价函数
def cost_function(X, y, weights, bias):
z = np.dot(X, weights) + bias
h = sigmoid(z)
J = (-1 / m) * np.sum(y * np.log(h) + (1 - y) * np.log(1 - h))
return J
# 主程序
def main():
filename = "data.txt"
data_x, data_y = load_data(filename)
# 初始化权重和偏置
initial_weights = np.zeros((data_x.shape[1], 1))
initial_bias = 0
# 设置学习率和迭代次数
learning_rate = 0.01
num_iterations = 1500
weights, bias, cost_history = gradient_descent(data_x, data_y, initial_weights, learning_rate, num_iterations)
# 绘制成本函数的变化
plt.plot(range(1, len(cost_history) + 1), cost_history, label='Cost')
plt.xlabel('Iterations')
plt.ylabel('Cost')
plt.title('Gradient Descent Learning Curve')
plt.legend()
plt.show()
if __name__ == "__main__":
main()
```
在这个例子中,我们首先加载数据,然后创建了一个Logistic回归模型,使用了梯度下降法优化权重和偏置。每一步迭代都会更新模型参数,并将每次迭代的损失记录下来,最后绘制出学习曲线。
阅读全文
相关推荐
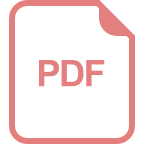
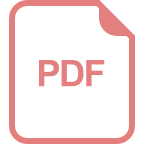
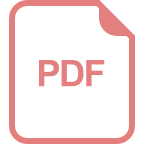


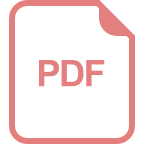
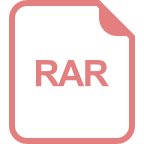
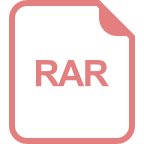
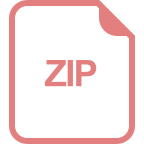
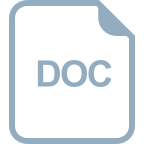
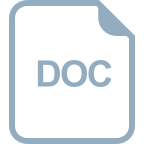
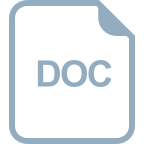
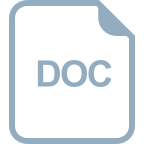
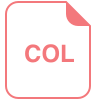
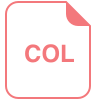
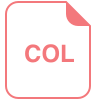
