使用cuda将摄像头读取的数据编码成h264流的代码
时间: 2024-03-05 07:49:51 浏览: 27
以下是使用CUDA将摄像头读取的数据编码成H264流的示例代码,使用了NVIDIA Video Codec SDK和OpenCV库:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
#include "NvEncoder/NvEncoderCuda.h"
using namespace std;
using namespace cv;
int main(int argc, char* argv[])
{
// 从摄像头读取数据
VideoCapture cap(0);
if (!cap.isOpened())
{
cout << "Failed to open camera!" << endl;
return -1;
}
// 设置编码器参数
int nWidth = 640, nHeight = 480;
int nBitrate = 1000000;
int nFps = 30;
int nGopSize = 30;
int nMaxConcurrentSessions = 1;
int nCodec = NV_ENC_H264;
std::string sPreset = "hq";
std::string sProfile = "high";
NvEncoderInitParam encodeParams = { 0 };
encodeParams.width = nWidth;
encodeParams.height = nHeight;
encodeParams.bitrate = nBitrate;
encodeParams.fps = nFps;
encodeParams.gopSize = nGopSize;
encodeParams.codec = nCodec;
encodeParams.preset = const_cast<char*>(sPreset.c_str());
encodeParams.profile = const_cast<char*>(sProfile.c_str());
encodeParams.maxConcurrentSessions = nMaxConcurrentSessions;
NvEncoderCuda enc(encodeParams);
// 分配编码器缓冲区
int nFrameSize = enc.GetFrameSize();
uint8_t* pFrame = new uint8_t[nFrameSize];
uint8_t* pBitstream = new uint8_t[nFrameSize];
// 编码并输出h264流
Mat frame;
while (true)
{
// 读取一帧图像
cap >> frame;
if (frame.empty())
{
break;
}
// 将帧数据复制到CUDA缓冲区
uint8_t* dpFrame = NULL;
int nPitch = 0;
enc.GetDeviceFrameBuffer(&dpFrame, &nPitch);
cudaMemcpy2D(dpFrame, nPitch, frame.data, frame.step, nWidth * 3, nHeight, cudaMemcpyHostToDevice);
// 编码一帧图像
int nBytes = 0;
enc.EncodeFrame(pFrame, &nBytes, dpFrame);
// 将编码后的数据复制回主机内存
cudaMemcpy(pBitstream, enc.GetBitstreamBuffer(), nBytes, cudaMemcpyDeviceToHost);
// 输出h264流
fwrite(pBitstream, sizeof(uint8_t), nBytes, stdout);
}
// 释放资源
enc.DestroyEncoder();
delete[] pFrame;
delete[] pBitstream;
return 0;
}
```
在编译时需要链接NVIDIA Video Codec SDK和OpenCV库。执行程序后,它将从摄像头读取数据并将其编码为H264流输出到标准输出。你可以将输出重定向到文件中,例如:
```
./encode | ffmpeg -i - output.mp4
```
这将从标准输入读取H264流,并将其转换为MP4文件。
相关推荐
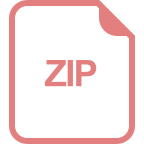














