NVIDIA CUDA 硬编码代码
时间: 2023-07-03 13:14:33 浏览: 349
好的,以下是一个简单的 NVIDIA CUDA 硬编码代码示例:
```cpp
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <cuda.h>
#include <cuda_runtime.h>
#include <nvcuvid.h>
#include <nvcuviddec.h>
#define CUDA_CHECK(call) \
do { \
CUresult status = call; \
if (status != CUDA_SUCCESS) { \
const char* err_msg; \
cuGetErrorString(status, &err_msg); \
fprintf(stderr, "CUDA error: %s\n", err_msg); \
exit(EXIT_FAILURE); \
} \
} while (0)
#define NVDEC_CHECK(call) \
do { \
NVDECSTATUS status = call; \
if (status != NVDEC_SUCCESS) { \
fprintf(stderr, "NVDEC error: %d\n", status); \
exit(EXIT_FAILURE); \
} \
} while (0)
int main(int argc, char** argv)
{
CUdevice cu_device;
CUcontext cu_ctx;
CUvideoctxlock cu_lock;
CUvideoparser cu_parser;
CUvideodecoder cu_decoder;
CUstream cu_stream;
CUdeviceptr cu_frame;
uint8_t* yuv_frame;
uint32_t frame_width, frame_height, frame_size;
uint32_t decoded_frames = 0;
// Initialize CUDA
CUDA_CHECK(cuInit(0));
CUDA_CHECK(cuDeviceGet(&cu_device, 0));
CUDA_CHECK(cuCtxCreate(&cu_ctx, 0, cu_device));
CUDA_CHECK(cuvidCtxLockCreate(&cu_lock, cu_ctx));
// Initialize decoder
NVDEC_CHECK(cuvidCreateVideoParser(&cu_parser, &nvcuvid_parser_params));
NVDEC_CHECK(cuvidCreateDecoder(&cu_decoder, &nvcuvid_dec_params));
// Initialize YUV frame buffer
frame_width = nvcuvid_dec_params.ulMaxWidth;
frame_height = nvcuvid_dec_params.ulMaxHeight;
frame_size = frame_width * frame_height * 3 / 2;
yuv_frame = (uint8_t*) malloc(frame_size);
CUDA_CHECK(cuMemAlloc(&cu_frame, frame_size));
// Initialize CUDA stream
CUDA_CHECK(cuStreamCreate(&cu_stream, 0));
// Loop over input frames
while (/* more input frames */) {
// Parse input frame
NVDEC_CHECK(cuvidParseVideoData(cu_parser, input_frame_data, input_frame_size, 0));
// Decode frame
CUVIDPICPARAMS pic_params = {0};
pic_params.CurrPicIdx = decoded_frames;
pic_params.ulTargetWidth = frame_width;
pic_params.ulTargetHeight = frame_height;
CUVIDPROCPARAMS proc_params = {0};
proc_params.progressive_frame = 1;
proc_params.second_field = 0;
proc_params.top_field_first = 1;
proc_params.unpaired_field = 1;
CUVIDGETDECODESTATUS status = {0};
NVDEC_CHECK(cuvidDecodePicture(cu_decoder, &pic_params));
NVDEC_CHECK(cuvidMapVideoFrame(cu_decoder, decoded_frames, &cu_frame, &frame_size, &proc_params));
NVDEC_CHECK(cuvidCtxLock(cu_lock, 0));
CUDA_CHECK(cuMemcpyDtoH(yuv_frame, cu_frame, frame_size));
NVDEC_CHECK(cuvidUnmapVideoFrame(cu_decoder, cu_frame));
NVDEC_CHECK(cuvidGetDecodeStatus(cu_decoder, decoded_frames, &status));
NVDEC_CHECK(cuvidCtxUnlock(cu_lock, 0));
// Process YUV frame
/* ... */
// Increment decoded frame count
decoded_frames++;
}
// Clean up
CUDA_CHECK(cuStreamSynchronize(cu_stream));
CUDA_CHECK(cuStreamDestroy(cu_stream));
CUDA_CHECK(cuMemFree(cu_frame));
free(yuv_frame);
NVDEC_CHECK(cuvidDestroyDecoder(cu_decoder));
NVDEC_CHECK(cuvidDestroyVideoParser(cu_parser));
cuvidCtxLockDestroy(cu_lock);
CUDA_CHECK(cuCtxDestroy(cu_ctx));
return 0;
}
```
注意,这只是一个简单的示例,实际使用中需要根据具体需求进行修改和优化。
阅读全文
相关推荐






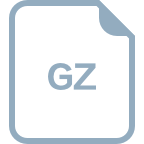








