C++打开一个文件夹下的所有文件
时间: 2023-12-19 21:03:51 浏览: 149
可以使用C++的文件操作库和递归函数来实现打开一个文件夹下的所有文件。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <dirent.h>
#include <cstring>
void scanDir(const char* path) {
DIR* dir = opendir(path);
if (dir == NULL) {
std::cerr << "Failed to open directory: " << path << std::endl;
return;
}
dirent* entry;
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) {
continue;
}
std::string fullPath = std::string(path) + "/" + entry->d_name;
if (entry->d_type == DT_DIR) {
// Recursively scan the subdirectory
scanDir(fullPath.c_str());
} else {
// Process the file
std::ifstream file(fullPath.c_str());
if (file.is_open()) {
std::cout << "Processing file: " << fullPath << std::endl;
// Do something with the file
file.close();
} else {
std::cerr << "Failed to open file: " << fullPath << std::endl;
}
}
}
closedir(dir);
}
int main() {
const char* path = "/path/to/directory";
scanDir(path);
return 0;
}
```
这个示例代码使用了C++的文件操作库和dirent.h头文件中的函数来实现递归遍历一个文件夹下的所有文件。在函数scanDir中,首先打开传入的文件夹,然后遍历文件夹中的所有文件和子文件夹。如果遍历到的是文件夹,则递归调用scanDir函数来处理子文件夹;如果遍历到的是文件,则打开文件并进行处理。
阅读全文
相关推荐
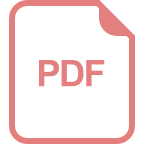
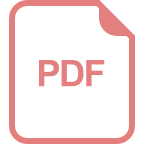
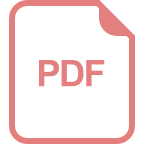
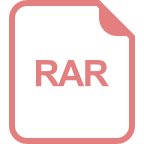
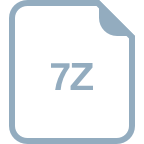
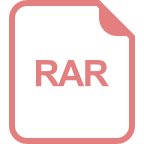
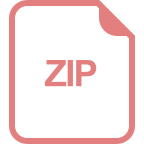
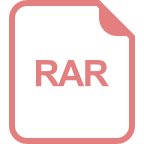
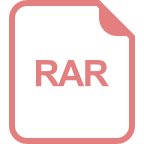
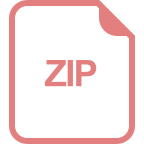
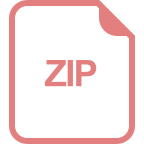
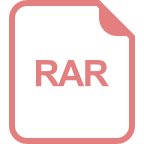
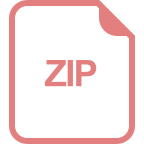
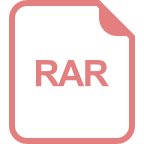
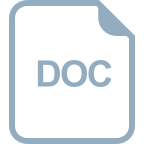
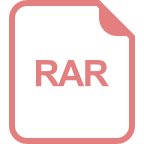