动态单链表查找第i个元素主函数代码
时间: 2023-06-01 07:02:58 浏览: 111
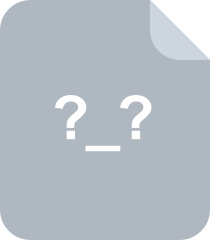
链表的主函数
以下是一个简单的动态单链表查找第i个元素的主函数代码示例:
```c++
#include <iostream>
using namespace std;
struct Node {
int data;
Node* next;
};
Node* head = NULL;
void insert(int value) {
Node* newNode = new Node;
newNode->data = value;
newNode->next = head;
head = newNode;
}
int search(int index) {
Node* current = head;
int count = 0;
while (current != NULL) {
if (count == index) {
return current->data;
}
count++;
current = current->next;
}
return -1; // Index out of bounds
}
int main() {
insert(5);
insert(10);
insert(15);
insert(20);
insert(25);
int index = 3;
int result = search(index);
if (result == -1) {
cout << "Index out of bounds" << endl;
} else {
cout << "Element at index " << index << " is " << result << endl;
}
return 0;
}
```
在上面的示例中,我们首先定义了一个`Node`结构体,其中包含一个整数`data`和一个指向下一个节点的指针`next`。然后,我们定义了一个全局指针`head`,表示动态单链表的头节点。
我们编写了一个名为`insert`的函数,用于在链表的头部插入新的节点。该函数接受一个整数参数`value`,创建一个新的节点,并将其插入到链表的头部。
然后,我们编写了一个名为`search`的函数,用于查找链表中的第i个元素。该函数接受一个整数参数`index`,表示要查找的元素的索引。函数遍历链表,每次遍历都将计数器`count`加1,直到找到第i个元素。在找到该元素后,函数返回该元素的值。
最后,在`main`函数中,我们向链表中插入了一些元素,然后调用`search`函数查找第3个元素。如果找到了该元素,则输出该元素的值。否则,输出“Index out of bounds”表示索引超出了链表的范围。
阅读全文
相关推荐




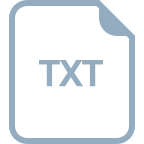
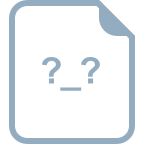
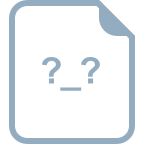
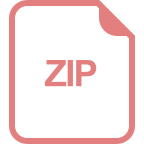
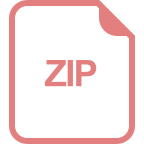
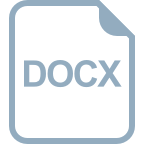
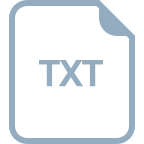




