单链表在第n个结点后插入数据为k的结点 用C语言写一个具体代码
时间: 2024-10-12 17:14:26 浏览: 36
单链表在第n个节点后插入新节点通常涉及两个步骤:首先找到第n个节点,然后在其后添加新的节点。这里有一个简单的C语言代码示例,假设我们已经有了链表结构`typedef struct Node`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点并插入到链表
void insertAfterNode(Node** head, int n, int k) {
if (*head == NULL) {
printf("List is empty.\n");
return;
}
// 遍历查找第n个节点
for (int i = 0; i < n - 1 && *head != NULL; i++) {
head = &(*head)->next;
}
// 如果找不到第n个节点,则表示n超出列表长度
if (*head == NULL) {
printf("Cannot find the node at position %d.\n", n);
return;
}
// 创建新节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = k;
// 插入新节点到链表
newNode->next = (*head)->next;
(*head)->next = newNode;
}
// 示例主函数
int main() {
// 初始化链表(这里仅作演示,实际链表需要自行构建)
Node* head = malloc(sizeof(Node));
head->data = 1;
head->next = NULL;
// 插入操作示例
insertAfterNode(&head, 2, 5); // 在第二个节点后插入值为5的新节点
// 打印链表验证结果
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
return 0;
}
```
这个代码会在`main`函数中创建一个包含单一元素1的简单链表,并尝试在第二个位置插入值为5的新节点。如果需要插入的位置不存在(即n大于链表长度),则会打印相应的错误信息。
阅读全文
相关推荐
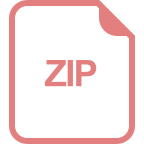
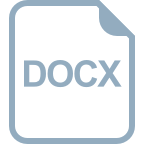
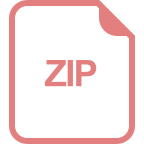
















