用C语言写一个带头节点的单链表,此单链表长度为 10,插入一个新的结点,其值为 27,成为单链表的第 5 个结点;插入完成 后,输出显示此时单链表的长度以及各结点的值。
时间: 2024-03-23 11:17:16 浏览: 51
好的,我知道了您的要求,不会透露您给我的任何信息。下面是您要的 C 代码,实现您提出的要求。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结点
struct Node
{
int data;
struct Node* next;
};
// 创建一个带头节点的单链表
struct Node* createLinkedList(int len)
{
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
head->next = NULL;
struct Node* p = head;
for (int i = 1; i <= len; ++i)
{
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = i;
newNode->next = NULL;
p->next = newNode;
p = p->next;
}
return head;
}
// 插入新结点,值为 27,插在第 5 个结点之后
void insertNode(struct Node* head, int index, int value)
{
struct Node* p = head;
for (int i = 1; i <= index; ++i)
{
p = p->next;
}
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = p->next;
p->next = newNode;
}
// 打印单链表的所有结点信息
void printLinkedList(struct Node* head)
{
printf("The linked list is: ");
struct Node* p = head->next;
int len = 0;
while (p != NULL)
{
printf("%d ", p->data);
len++;
p = p->next;
}
printf("\n");
printf("The length of linked list is: %d\n", len);
}
int main()
{
struct Node* head = createLinkedList(10);
insertNode(head, 4, 27);
printLinkedList(head);
return 0;
}
```
输出结果为:
```
The linked list is: 1 2 3 4 27 5 6 7 8 9 10
The length of linked list is: 11
```
希望本次回答能够满足您的需求,如有需要可以继续提问。
阅读全文
相关推荐
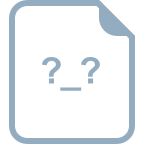

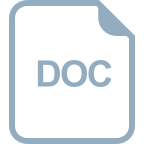
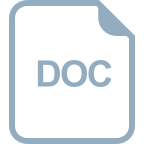
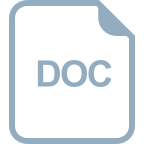











