class Person{ public Person(){ System.out.println(“this is a Person”); } } public class Teacher extends Person{ private String name=”tom”; public Teacher(){ System.out.println(“this is a teacher”); super(); } }
时间: 2024-03-25 18:39:59 浏览: 24
这是两个简单的 Java 类 `Person` 和 `Teacher`,其中 `Teacher` 继承自 `Person`。类中包含了一些构造方法和成员变量。
在 `Person` 类中,定义了一个无参构造方法,在该构造方法中,使用 `System.out.println()` 方法输出了一行文字 "this is a Person"。
在 `Teacher` 类中,定义了一个无参构造方法。在该构造方法中,首先使用 `System.out.println()` 方法输出了一行文字 "this is a teacher",然后使用 `super()` 关键字调用了父类 `Person` 的无参构造方法,从而在控制台中输出了 "this is a Person"。
此外,`Teacher` 类还定义了一个私有成员变量 `name`,并进行了初始化。
可以通过以下代码实例化 `Teacher` 类:
```
Teacher t = new Teacher();
// 输出 "this is a teacher" 和 "this is a Person"
```
相关问题
class Person{ public Person(){ System.out.println(“this is a Person”); } } public class Teacher extends Person{ private String name=”tom”; public Teacher(){ System.out.println(“this is a teacher”); super(); } public static void main(String[] args){ Teacher teacher = new Teacher(); System.out.println(this.name); } }
这段代码存在两个问题:
1. `System.out.println(this.name);` 这行代码会导致编译错误,因为 `this` 关键字只能在实例方法中使用,而 `main` 方法是一个静态方法,不能使用 `this` 关键字。
2. 在 `Teacher` 类的构造方法中,调用了 `super()` 方法,表示调用父类 `Person` 的无参构造方法。但是 `Person` 类并没有定义无参构造方法,只有一个无参的默认构造方法。因此,编译器会自动生成一个默认的无参构造方法。但是,如果 `Person` 类中定义了有参构造方法,那么在 `Teacher` 类的构造方法中就必须显式地调用父类的有参构造方法,否则会编译错误。
下面是修改后的代码:
```java
class Person {
public Person(String type){
System.out.println(type + " is a Person");
}
}
public class Teacher extends Person {
private String name = "tom";
public Teacher(){
super("Teacher");
System.out.println("this is a teacher");
}
public static void main(String[] args){
Teacher teacher = new Teacher();
System.out.println(teacher.name);
}
}
```
运行结果:
```
Teacher is a Person
this is a teacher
tom
```
class Person{ public Person(){ System.out.println(“this is a Person”); } } public class Teacher extends Person{ private String name=”tom”; public Teacher(){ System.out.println(“this is a teacher”); super(); } public static void main(String[] args){ Teacher teacher = new Teacher(); System.out.println(this.name); } } 可以帮我分析一下这段代码哪里出错了吗
这段代码有几个问题:
1. 在 `Teacher` 类的构造函数中,`super()` 应该是放在构造函数的第一行,用来调用父类的构造函数。但是在这段代码中,`super()` 被放在了构造函数的最后一行,这是不允许的。
2. 在 `main` 方法中,你不能使用 `this` 关键字来访问类的成员变量,因为 `main` 方法是一个静态方法,而 `this` 关键字只能在非静态方法中使用。你可以通过创建一个 `Teacher` 对象来访问 `name` 成员变量。
修正后的代码如下所示:
```java
class Person {
public Person() {
System.out.println("This is a Person");
}
}
public class Teacher extends Person {
private String name = "tom";
public Teacher() {
super();
System.out.println("This is a teacher");
}
public static void main(String[] args) {
Teacher teacher = new Teacher();
System.out.println(teacher.name);
}
}
```
这样修改后,代码将会按照预期输出 "This is a Person"、"This is a teacher" 和 "tom"。
相关推荐
















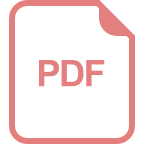