分析下列Java程序,判断这个程序输出什么,并分析为什么输出这个结果class Person{public Person(){ System.out.println("this is a Person");}}public class Teacher extends Person{private String name="tom";public Teacher(){super();System.out.println("this is a Teacher");this.name="kate";}public static void main(String[] args){Teacher teacher = new Teacher();System.out.println(teacher.name);}}
时间: 2024-02-25 13:59:27 浏览: 16
这个Java程序输出:
```
this is a Person
this is a Teacher
kate
```
这个输出结果的原因是:
1. 当Teacher类被创建时,它继承了Person类,因此Person类的构造函数被自动调用,并输出 "this is a Person"。
2. Teacher类的构造函数被调用,它首先调用它的父类构造函数(即Person类的构造函数)通过super()来实现,并输出 "this is a Teacher"。
3. Teacher类的构造函数设置了它的私有变量name的值为"kate"。
4. main函数创建了一个Teacher对象,并输出teacher对象的name变量,即输出"kate"。
相关问题
java创建2个person对象,给对象的属性赋值,并输出年龄较大的那个person的姓名
首先,我们需要创建一个`Person`类来表示一个人的属性和行为。该类应该包含姓名和年龄两个属性,并提供方法来获取和设置这些属性值。然后,我们可以创建两个`Person`对象,并为每个对象的姓名和年龄属性赋值。最后,我们比较两个对象的年龄并输出年龄较大的那个人的姓名。
```java
// 定义Person类
class Person {
private String name;
private int age;
// 构造方法
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// 获取姓名
public String getName() {
return name;
}
// 获取年龄
public int getAge() {
return age;
}
}
// 主类
public class Main {
public static void main(String[] args) {
// 创建两个Person对象并赋值
Person person1 = new Person("张三", 25);
Person person2 = new Person("李四", 30);
// 比较两个对象的年龄并输出年龄较大的那个人的姓名
if (person1.getAge() > person2.getAge()) {
System.out.println("年龄较大的人是:" + person1.getName());
} else {
System.out.println("年龄较大的人是:" + person2.getName());
}
}
}
```
以上代码创建了两个`Person`对象,分别为`person1`和`person2`,并赋予了姓名和年龄属性的值。然后,通过比较两个对象的年龄,输出了年龄较大的那个人的姓名。在这个示例中,输出结果为"年龄较大的人是:李四",因为李四的年龄为30,大于张三的年龄25。
class Person{ public Person(){ System.out.println(“this is a Person”); } }
这是一个简单的 Java 类 `Person`,包含一个无参构造方法。在该构造方法中,使用 `System.out.println()` 方法输出了一行文字 "this is a Person"。
该类可以被实例化,每次实例化时都会自动执行该构造方法,并输出 "this is a Person"。
可以通过以下代码实例化该类:
```
Person p = new Person(); // 输出 "this is a Person"
```
相关推荐
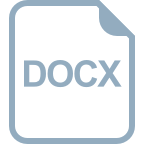
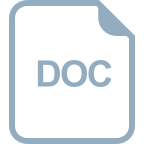
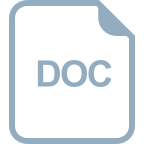












