请编写程序实现链表的建立、插入、删除、遍历的基本运算,其中顺序表中每一个数据元素的类型定义为学生信息的结构体,包括学号、姓名和成绩。
时间: 2023-05-11 16:04:52 浏览: 41
好的,以下是链表的建立、插入、删除、遍历的基本运算的程序实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 学生信息结构体
typedef struct student {
int id; // 学号
char name[20]; // 姓名
int score; // 成绩
struct student *next; // 指向下一个节点的指针
} Student;
// 创建一个新的节点
Student *create_node(int id, char *name, int score) {
Student *node = (Student *)malloc(sizeof(Student));
node->id = id;
strcpy(node->name, name);
node->score = score;
node->next = NULL;
return node;
}
// 在链表末尾插入一个节点
void insert_node(Student **head, Student *node) {
if (*head == NULL) {
*head = node;
} else {
Student *p = *head;
while (p->next != NULL) {
p = p->next;
}
p->next = node;
}
}
// 删除指定学号的节点
void delete_node(Student **head, int id) {
if (*head == NULL) {
return;
}
if ((*head)->id == id) {
Student *p = *head;
*head = (*head)->next;
free(p);
} else {
Student *p = *head;
while (p->next != NULL && p->next->id != id) {
p = p->next;
}
if (p->next != NULL) {
Student *q = p->next;
p->next = q->next;
free(q);
}
}
}
// 遍历链表并输出每个节点的信息
void traverse_list(Student *head) {
if (head == NULL) {
printf("链表为空!\n");
} else {
printf("学号\t姓名\t成绩\n");
Student *p = head;
while (p != NULL) {
printf("%d\t%s\t%d\n", p->id, p->name, p->score);
p = p->next;
}
}
}
int main() {
Student *head = NULL;
int choice, id, score;
char name[20];
while (1) {
printf("\n1. 插入节点\n");
printf("2. 删除节点\n");
printf("3. 遍历链表\n");
printf("4. 退出程序\n");
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入学号、姓名和成绩:");
scanf("%d%s%d", &id, name, &score);
insert_node(&head, create_node(id, name, score));
break;
case 2:
printf("请输入要删除的学号:");
scanf("%d", &id);
delete_node(&head, id);
break;
case 3:
traverse_list(head);
break;
case 4:
exit(0);
default:
printf("无效的选择!\n");
}
}
return 0;
}
```
希望这个程序能够帮助你实现链表的基本运算。如果你有任何问题,请随时问我。
相关推荐
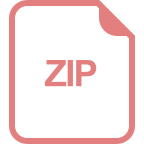
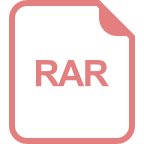
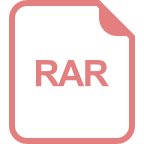








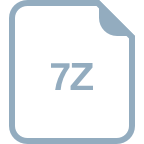
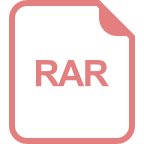
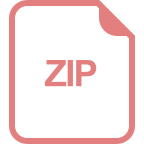
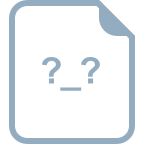
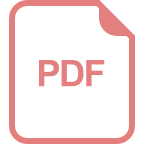
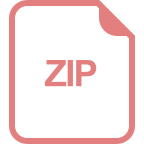
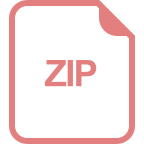