Verilog HDL使用状态机实现DS18B20工作逻辑
时间: 2024-01-19 20:43:16 浏览: 211
DS18B20是一种数字温度传感器,其通信协议比较特殊,需要使用状态机来实现其工作逻辑。下面是一个基于Verilog HDL的DS18B20状态机实现:
```verilog
module ds18b20(
input clk, // 时钟信号
input rst, // 复位信号
input start, // 启动传输信号
output reg dq // 数据线信号
);
// 定义状态机状态
localparam IDLE = 2'b00;
localparam RESET = 2'b01;
localparam START_CONV = 2'b10;
localparam READ_SCRATCHPAD = 2'b11;
// 定义状态机寄存器
reg [1:0] state_reg, state_next;
reg [3:0] cnt_reg, cnt_next;
reg [7:0] scratchpad_reg, scratchpad_next;
// 初始化寄存器
initial begin
state_reg = IDLE;
cnt_reg = 0;
scratchpad_reg = 0;
end
// 定义状态机转移逻辑
always @ (posedge clk or posedge rst) begin
if (rst) begin
state_reg <= IDLE;
cnt_reg <= 0;
scratchpad_reg <= 0;
end else begin
state_reg <= state_next;
cnt_reg <= cnt_next;
scratchpad_reg <= scratchpad_next;
end
end
// 定义状态机行为逻辑
always @ (*) begin
state_next = state_reg;
cnt_next = cnt_reg;
scratchpad_next = scratchpad_reg;
dq = 1;
case (state_reg)
IDLE: begin
cnt_next = 0;
if (start) begin
state_next = RESET;
end
end
RESET: begin
cnt_next = cnt_reg + 1;
dq = 0;
if (cnt_reg >= 480) begin
state_next = START_CONV;
end
end
START_CONV: begin
cnt_next = cnt_reg + 1;
dq = 0;
if (cnt_reg >= 12) begin
state_next = READ_SCRATCHPAD;
end
end
READ_SCRATCHPAD: begin
cnt_next = cnt_reg + 1;
dq = 0;
if (cnt_reg >= 80) begin
scratchpad_next = {dq, scratchpad_reg[7:1]};
cnt_next = 1;
state_next = READ_SCRATCHPAD;
end else if (cnt_reg >= 16) begin
dq = 1;
end else if (cnt_reg >= 8) begin
dq = scratchpad_reg[0];
end
end
endcase
end
endmodule
```
在这个Verilog HDL代码中,我们定义了一个包含4个状态的状态机,分别是IDLE、RESET、START_CONV和READ_SCRATCHPAD。其中,IDLE状态表示处于空闲状态,等待启动传输信号;RESET状态表示发送复位信号;START_CONV状态表示发送启动温度转换命令;READ_SCRATCHPAD状态表示读取温度数据。
在状态机的行为逻辑中,我们根据DS18B20通信协议的时序要求,控制数据线dq的输出,以及状态机的状态转移。其中,我们使用计数器cnt来控制时序,使用scratchpad_reg来存储读取到的温度数据。最终,我们可以将这个状态机与其他模块组合起来,实现完整的DS18B20数字温度传感器。
阅读全文
相关推荐
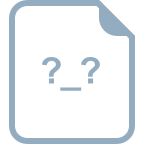

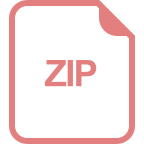
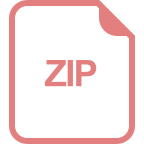
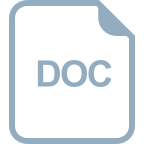
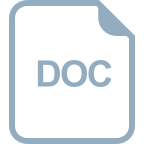


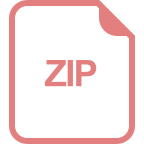
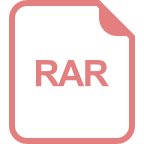
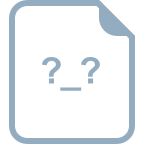
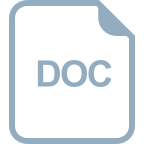
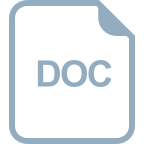
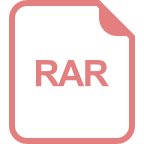
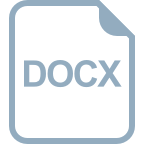