modbus rtu 程序C代码
时间: 2024-05-26 08:06:33 浏览: 20
Modbus RTU是一种常用的串行通信协议,用于在工业自动化领域中实现设备之间的通信。下面是一个简单的Modbus RTU程序的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define DEVICE "/dev/ttyUSB0" // 串口设备路径
#define BAUDRATE B9600 // 波特率
#define SLAVE_ID 1 // 从机ID
int main() {
int fd;
struct termios options;
// 打开串口设备
fd = open(DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("无法打开串口设备");
return -1;
}
// 配置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, BAUDRATE);
cfsetospeed(&options, BAUDRATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 发送Modbus RTU请求
unsigned char request[8] = {SLAVE_ID, 0x03, 0x00, 0x00, 0x00, 0x01, 0x84, 0x0A};
write(fd, request, sizeof(request));
// 读取Modbus RTU响应
unsigned char response[256];
int numBytes = read(fd, response, sizeof(response));
if (numBytes < 0) {
perror("读取串口数据失败");
return -1;
}
// 处理Modbus RTU响应
if (response[0] == SLAVE_ID && response[1] == 0x03 && response[2] == 0x02) {
int value = (response[3] << 8) | response[4];
printf("读取到的值为:%d\n", value);
} else {
printf("Mod RTU响应错误\n");
}
// 关闭串口设备
close(fd);
return 0;
}
```
这段代码实现了一个简单的Modbus RTU主机程序,它通过串口与从机通信,并读取从机的寄存器值。你可以根据实际需求进行修改和扩展。
相关推荐
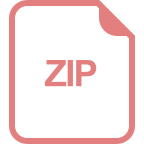
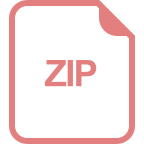














