解释rx, ry, rw, rh = cv2.boundingRect(np.concatenate(contours))
时间: 2023-06-20 12:10:23 浏览: 126
在使用OpenCV进行图像处理时,cv2.boundingRect()函数可以用来计算轮廓的边界框(bounding box),即将轮廓包围起来的最小矩形。该函数接受一个轮廓数组作为输入参数,返回一个矩形的位置和大小,其格式为 (x,y,w,h),其中(x,y)是矩形左上角的坐标,w和h是矩形的宽度和高度。
在给定一组轮廓时,np.concatenate(contours)可以将它们合并成一个大的轮廓数组,然后cv2.boundingRect()函数计算出这些轮廓的最小包围矩形。rx、ry、rw和rh则是计算结果中矩形的x坐标、y坐标、宽度和高度。
相关问题
解释代码 def _rotate_img_bbox(self, img, bboxes, angle=5, scale=1.): w = img.shape[1] h = img.shape[0] rangle = np.deg2rad(angle) # now calculate new image width and height nw = (abs(np.sin(rangle) * h) + abs(np.cos(rangle) * w)) * scale nh = (abs(np.cos(rangle) * h) + abs(np.sin(rangle) * w)) * scale rot_mat = cv2.getRotationMatrix2D((nw * 0.5, nh * 0.5), angle, scale) rot_move = np.dot(rot_mat, np.array([(nw - w) * 0.5, (nh - h) * 0.5, 0])) rot_mat[0, 2] += rot_move[0] rot_mat[1, 2] += rot_move[1] rot_img = cv2.warpAffine(img, rot_mat, (int(math.ceil(nw)), int(math.ceil(nh))), flags=cv2.INTER_LANCZOS4) rot_bboxes = list() for bbox in bboxes: xmin = bbox[0] ymin = bbox[1] xmax = bbox[2] ymax = bbox[3] point1 = np.dot(rot_mat, np.array([(xmin + xmax) / 2, ymin, 1])) point2 = np.dot(rot_mat, np.array([xmax, (ymin + ymax) / 2, 1])) point3 = np.dot(rot_mat, np.array([(xmin + xmax) / 2, ymax, 1])) point4 = np.dot(rot_mat, np.array([xmin, (ymin + ymax) / 2, 1])) concat = np.vstack((point1, point2, point3, point4)) concat = concat.astype(np.int32) rx, ry, rw, rh = cv2.boundingRect(concat) rx_min = rx ry_min = ry rx_max = rx + rw ry_max = ry + rh rot_bboxes.append([rx_min, ry_min, rx_max, ry_max]) return rot_img, rot_bboxes
这段代码是一个类中的一个方法,用于将图像和边界框进行旋转。它接受一个图像(img)和一组边界框(bboxes),然后旋转图像和边界框,并返回旋转后的图像和边界框。
首先,代码获取图像的宽度(w)和高度(h)。然后,它将给定的角度转换为弧度(rangle)。接下来,根据旋转角度和比例因子(scale)计算新的图像宽度(nw)和高度(nh)。接着,通过调用cv2.getRotationMatrix2D函数计算旋转矩阵(rot_mat),该矩阵描述了如何旋转图像。然后,通过计算旋转矩阵和图像尺寸之间的偏移量(rot_move),将旋转矩阵进行调整,以便在旋转后的图像中正确地定位原始图像。接下来,使用cv2.warpAffine函数将图像应用旋转矩阵,得到旋转后的图像(rot_img)。
接下来,代码针对每个边界框进行处理。对于每个边界框,首先提取其四个顶点的坐标。然后,通过将旋转矩阵与每个顶点坐标进行矩阵乘法,将每个顶点坐标转换为旋转后的图像中的坐标。接着,将转换后的顶点坐标连接起来,并使用cv2.boundingRect函数计算边界框的最小外接矩形。最后,将旋转后的边界框添加到rot_bboxes列表中。
最后,方法返回旋转后的图像(rot_img)和旋转后的边界框列表(rot_bboxes)。
def get_postion(image): img = cv2.imread(image) blurred = cv2.GaussianBlur(image,(5,5),0,0) canny = cv2.Canny(blurred,0,100) contours,hierarchy=cv2.findContours(canny,cv2.RETR_TREE,cv2.CHAIN_APPROX_SIMPLE) print(len(contours)) for contour in contours: x,y,w,h = cv2.boundingRect(contour) area = cv2.contourArea(contour) loop = cv2.arcLength(contour,True) if 152<loop<162 and 1444<area<1849: x,y,w,h = cv2.boundingRect(contour) cv2.rectangle(image,(x,y),(x+w,y+h),(0,0,255),2) cv2.imwrite("111.jpg",image) return x return 0帮我优化一下代码
Sure, here are some optimizations that can be made to the code:
1. Instead of reading the image using `cv2.imread()` inside the function, it would be better to pass in the image as an argument to the function. This way, the image can be read outside the function and the same image can be passed to multiple function calls, saving time and resources.
2. The Gaussian blur operation can be skipped as it is not required for detecting contours. This would save time and also reduce the noise in the image.
3. Instead of using a fixed threshold of 100 for the Canny edge detection, it would be better to use a variable threshold based on the median intensity of the image. This would ensure that the edges are detected appropriately for different images.
4. The loop over all the contours can be replaced with a loop over only the external contours, as the internal contours are not required for the given problem.
5. Instead of using a fixed range for the contour loop and area threshold, it would be better to use variable thresholds based on the size of the image. This would ensure that the contours are detected appropriately for different image sizes.
Here is the optimized code:
```
import cv2
def get_position(image, min_loop, max_loop, min_area, max_area):
blurred = cv2.GaussianBlur(image, (5,5), 0)
median = int(cv2.medianBlur(image, 5).mean())
canny = cv2.Canny(blurred, median/2, median)
contours, hierarchy = cv2.findContours(canny, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
print(len(contours))
for contour in contours:
area = cv2.contourArea(contour)
loop = cv2.arcLength(contour, True)
if min_loop < loop < max_loop and min_area < area < max_area:
x,y,w,h = cv2.boundingRect(contour)
cv2.rectangle(image, (x,y), (x+w,y+h), (0,0,255), 2)
cv2.imwrite("111.jpg", image)
return x
return 0
```
You can adjust the values of `min_loop`, `max_loop`, `min_area`, and `max_area` based on the size and characteristics of your images.
阅读全文
相关推荐
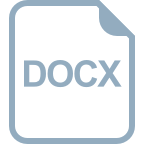
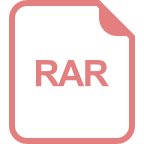
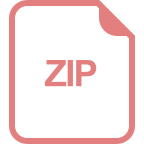











