import java.util.Scanner; public class Main { public static void main(String[] args) { int[] ary = new int[6]; int max; int min; Scanner scanner = new Scanner(System.in); for (int i = 0; i <ary.length ; i++) { ary[i]=scanner.nextInt(); } //write your code here...... max = ary[0]; min = ary[0]; for(int i = 0 ; i < ary.length ; i++){ if(max <+ ary[i]){ max = ary[i]; } } for(int i = 0 ; i < ary.length ; i++){ if(min >= ary[i]){ min = ary[i]; } } Arrays.sort(ary); max = ary[5]; min = ary[0]; System.out.println(max+" "+min); } }
时间: 2024-02-14 20:32:01 浏览: 65
这段代码是用来输入6个整数,并找出其中的最大值和最小值。代码逻辑如下:
1. 创建一个大小为6的整型数组ary和一个Scanner对象scanner来读取输入。
2. 使用for循环遍历数组ary,将用户输入的整数存储在数组中。
3. 初始化max和min变量为数组ary的第一个元素。
4. 使用for循环遍历数组ary,找出最大值max和最小值min。
5. 使用Arrays.sort()方法对数组ary进行排序。
6. 更新max和min变量为排序后的数组ary的最后一个元素和第一个元素。
7. 打印出最大值和最小值。
如果你有其他问题,可以继续提问。
相关问题
改正以下代码 import java.util.Scanner; public class Lab { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); int tempInt; double output; tempInt = scnr.nextInt(); double output = (double) tempInt; System.out.println(output); } }
以下是改正后的代码:
import java.util.Scanner;
public class Lab {
public static void main(String args[]) {
Scanner scnr = new Scanner(System.in);
int tempInt;
double output;
tempInt = scnr.nextInt();
output = (double) tempInt;
System.out.println(output);
}
}
问题在于第7行,double output = (double) tempInt; 中的double output 是重复声明了一个变量,需要删除。
import java.math.BigInteger; import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int T = sc.nextInt(); while (T-- != 0) { int a = sc.nextInt(); int b = sc.nextInt(); int c = sc.nextInt(); for(int i = 0 ; i < c ; i ++) { double num = Math.pow(2,i) * b + num; System.out.println(num + a); } } } }帮我改正这个java代码
很高兴能帮上忙!改正过的代码如下:import java.math.BigInteger;
import java.util.Scanner;public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int T = sc.nextInt();
while (T-- != 0) {
int a = sc.nextInt();
int b = sc.nextInt();
int c = sc.nextInt();
for(int i = 0 ; i < c ; i++) {
double num = Math.pow(2,i) * b;
System.out.println(num + a);
}
}
}
}
阅读全文
相关推荐
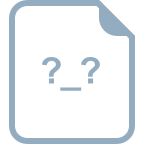





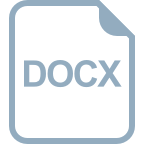
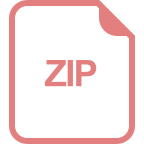
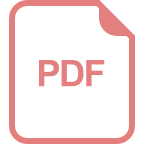
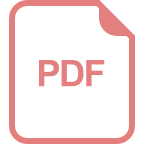
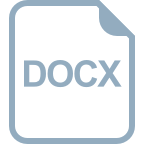
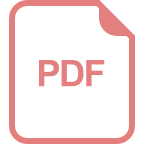