设计一个二维矩阵类 ,成员变量为double*类型,用来存储矩阵中的元素 ,写出赋值运算符重载(包括+=,-=,*=),拷贝构造函数和构造函数
时间: 2024-03-22 21:41:20 浏览: 18
好的,我可以帮你设计一个二维矩阵类。以下是该类的定义:
```cpp
class Matrix {
public:
Matrix(int rows, int cols); // 构造函数
Matrix(const Matrix& other); // 拷贝构造函数
~Matrix(); // 析构函数
// 重载赋值运算符
Matrix& operator=(const Matrix& other);
Matrix& operator+=(const Matrix& other);
Matrix& operator-=(const Matrix& other);
Matrix& operator*=(double scalar);
// 获取矩阵元素
double& operator()(int row, int col);
const double& operator()(int row, int col) const;
// 获取矩阵行数和列数
int rows() const;
int cols() const;
private:
int m_rows; // 矩阵行数
int m_cols; // 矩阵列数
double* m_data; // 存储矩阵元素的指针
};
```
其中,构造函数和析构函数的实现如下:
```cpp
Matrix::Matrix(int rows, int cols) : m_rows(rows), m_cols(cols), m_data(new double[rows * cols]) {}
Matrix::Matrix(const Matrix& other) : m_rows(other.m_rows), m_cols(other.m_cols), m_data(new double[other.m_rows * other.m_cols]) {
std::memcpy(m_data, other.m_data, m_rows * m_cols * sizeof(double));
}
Matrix::~Matrix() {
delete[] m_data;
}
```
重载赋值运算符的实现如下:
```cpp
Matrix& Matrix::operator=(const Matrix& other) {
if (this != &other) {
delete[] m_data;
m_rows = other.m_rows;
m_cols = other.m_cols;
m_data = new double[m_rows * m_cols];
std::memcpy(m_data, other.m_data, m_rows * m_cols * sizeof(double));
}
return *this;
}
Matrix& Matrix::operator+=(const Matrix& other) {
assert(m_rows == other.m_rows && m_cols == other.m_cols);
for (int i = 0; i < m_rows * m_cols; ++i) {
m_data[i] += other.m_data[i];
}
return *this;
}
Matrix& Matrix::operator-=(const Matrix& other) {
assert(m_rows == other.m_rows && m_cols == other.m_cols);
for (int i = 0; i < m_rows * m_cols; ++i) {
m_data[i] -= other.m_data[i];
}
return *this;
}
Matrix& Matrix::operator*=(double scalar) {
for (int i = 0; i < m_rows * m_cols; ++i) {
m_data[i] *= scalar;
}
return *this;
}
```
获取矩阵元素的实现如下:
```cpp
double& Matrix::operator()(int row, int col) {
assert(row >= 0 && row < m_rows && col >= 0 && col < m_cols);
return m_data[row * m_cols + col];
}
const double& Matrix::operator()(int row, int col) const {
assert(row >= 0 && row < m_rows && col >= 0 && col < m_cols);
return m_data[row * m_cols + col];
}
```
获取矩阵行数和列数的实现如下:
```cpp
int Matrix::rows() const {
return m_rows;
}
int Matrix::cols() const {
return m_cols;
}
```
希望这个二维矩阵类能够满足你的需求。
相关推荐
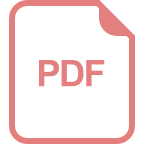
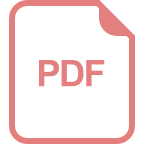
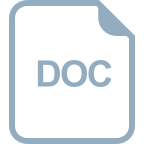














