MapReduce使用快速排序算法实现对文件里面数字进行排序的java代码
时间: 2023-07-18 10:20:52 浏览: 111
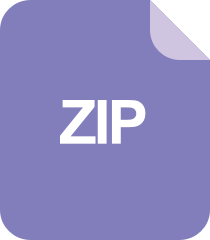
All Algorithms implemented in Java.zip
下面是使用MapReduce实现对文件里面数字进行排序的Java代码,其中使用了快速排序算法:
```java
import java.io.IOException;
import java.util.*;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class SortNumbers {
public static class Map extends Mapper<LongWritable, Text, IntWritable, NullWritable> {
private IntWritable num = new IntWritable();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
StringTokenizer tokenizer = new StringTokenizer(line);
while (tokenizer.hasMoreTokens()) {
num.set(Integer.parseInt(tokenizer.nextToken()));
context.write(num, NullWritable.get());
}
}
}
public static class Reduce extends Reducer<IntWritable, NullWritable, IntWritable, NullWritable> {
public void reduce(IntWritable key, Iterable<NullWritable> values, Context context) throws IOException, InterruptedException {
context.write(key, NullWritable.get());
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "sort numbers");
job.setJarByClass(SortNumbers.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(IntWritable.class);
job.setOutputValueClass(NullWritable.class);
job.setNumReduceTasks(1); // 设置Reduce任务数为1
// 设置输入和输出文件路径
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在这段代码中,Map任务将输入文件按照空格分隔符进行拆分,将每个数字作为key值输出,value值为NullWritable。Reduce任务将输入的key值进行全局排序,并输出结果。最终结果会按照升序排列的顺序输出到输出文件中。
阅读全文
相关推荐
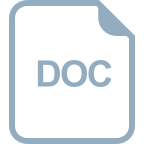
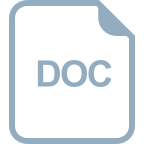
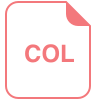
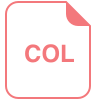
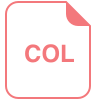
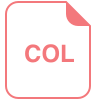

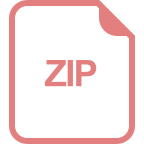
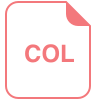
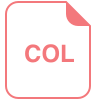
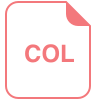
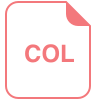
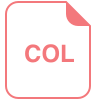
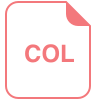
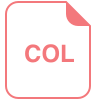
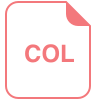
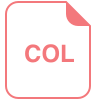