用Java实现中缀表达式转后缀表达式 包含负号和左括号
时间: 2024-02-11 14:06:57 浏览: 82
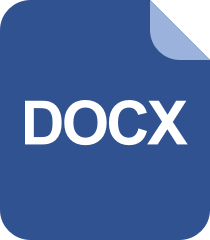
Java中缀表达式求值

好的,这个问题我可以帮你回答。中缀表达式转后缀表达式可以使用栈来实现。具体的步骤如下:
1. 创建一个栈和一个存储后缀表达式的字符串变量。
2. 从左到右遍历中缀表达式的每个字符。
3. 如果当前字符是数字或者小数点,直接将其添加到后缀表达式字符串中。
4. 如果当前字符是左括号,将其压入栈中。
5. 如果当前字符是右括号,将栈中的元素依次弹出并添加到后缀表达式字符串中,直到遇到左括号为止。注意:左括号不需要添加到后缀表达式字符串中。
6. 如果当前字符是运算符,判断其和栈顶运算符的优先级,如果栈顶运算符优先级高于或等于当前运算符,则将栈顶运算符弹出并添加到后缀表达式字符串中,直到栈为空或者栈顶运算符的优先级低于当前运算符。然后将当前运算符压入栈中。
7. 如果当前字符是负号,需要判断其是负数还是减号。如果负号前面是左括号或者是表达式的开头,说明是负数,将其视为一个整体添加到后缀表达式字符串中;否则是减号,按照运算符处理。
8. 重复步骤2-7直到遍历完中缀表达式。
9. 将栈中的元素依次弹出并添加到后缀表达式字符串中。
10. 返回后缀表达式字符串。
下面是一个用Java实现的示例代码,你可以参考一下:
```java
public static String infixToPostfix(String infix) {
Stack<Character> stack = new Stack<>();
StringBuilder postfix = new StringBuilder();
for (int i = 0; i < infix.length(); i++) {
char ch = infix.charAt(i);
if (Character.isDigit(ch) || ch == '.') {
postfix.append(ch);
} else if (ch == '(') {
stack.push(ch);
} else if (ch == ')') {
while (!stack.isEmpty() && stack.peek() != '(') {
postfix.append(stack.pop());
}
stack.pop(); // 弹出左括号
} else if (isOperator(ch)) {
while (!stack.isEmpty() && getPriority(stack.peek()) >= getPriority(ch)) {
postfix.append(stack.pop());
}
stack.push(ch);
} else if (ch == '-') {
if (i == 0 || infix.charAt(i - 1) == '(') { // 负数的情况
postfix.append('-');
i++;
while (i < infix.length() && (Character.isDigit(infix.charAt(i)) || infix.charAt(i) == '.')) {
postfix.append(infix.charAt(i));
i++;
}
i--;
} else { // 减号的情况
while (!stack.isEmpty() && getPriority(stack.peek()) >= getPriority(ch)) {
postfix.append(stack.pop());
}
stack.push(ch);
}
}
}
while (!stack.isEmpty()) {
postfix.append(stack.pop());
}
return postfix.toString();
}
private static boolean isOperator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
private static int getPriority(char ch) {
if (ch == '+' || ch == '-') {
return 1;
} else if (ch == '*' || ch == '/') {
return 2;
} else {
return 0;
}
}
```
阅读全文
相关推荐
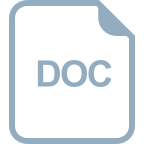
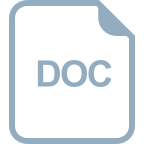
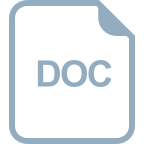
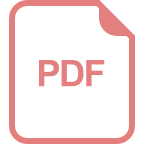


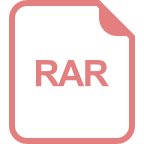
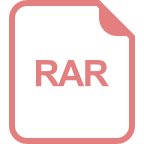
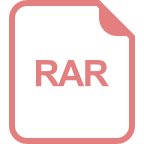
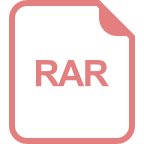
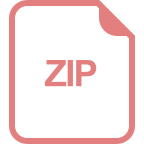
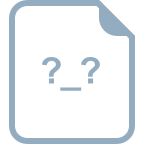

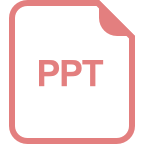
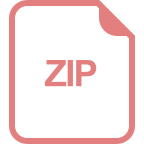
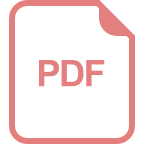
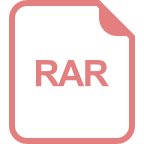
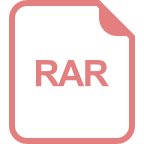