python3 datetime 减少一秒钟
时间: 2024-07-15 22:00:19 浏览: 63
在Python 3中,`datetime`模块提供了一种方便的方式来处理日期和时间。如果你想要减少一个日期或时间对象的一秒钟,你可以使用`timedelta`对象,它是时间间隔的表示。以下是如何减去一秒的示例:
```python
from datetime import datetime, timedelta
# 假设你有一个datetime对象
current_time = datetime.now()
# 减去1秒
new_time = current_time - timedelta(seconds=1)
print("原时间:", current_time)
print("减一秒后的时间:", new_time)
```
运行这段代码后,`new_time` 就会是比 `current_time` 少一秒钟的时间。
相关问题
python datetime 减少一秒钟
在Python中,如果你有一个`datetime`对象并且想要减少一秒,你可以直接调用`replace()`方法并传入`seconds`参数减一。这里是一个例子:
```python
from datetime import datetime, timedelta
# 假设你有一个datetime对象
current_time = datetime.now()
# 减少一秒
new_time = current_time.replace(seconds=current_time.second - 1)
print(new_time)
```
如果你想要确保秒数不会小于0,可以在减一之前检查当前秒数,如果已经是0,则保持不变:
```python
if current_time.second > 0:
new_time = current_time.replace(seconds=current_time.second - 1)
else:
new_time = current_time
print(new_time)
```
datetime.datetime用什么引用时间间隔
`datetime.datetime` 是 Python 中处理日期和时间的标准库,它可以用来表示特定的日期和时间。在创建 `datetime.datetime` 对象时,可以用不同的参数指定日期和时间的细节,其中包括年、月、日、时、分、秒等。
在 `datetime.datetime` 中还有一个重要的概念是时间间隔,可以使用 `datetime.timedelta` 类来表示时间间隔。可以将 `datetime.timedelta` 对象添加到 `datetime.datetime` 对象中,从而得到新的日期和时间。
`datetime.timedelta` 对象可以通过以下方式创建:
```python
import datetime
# 创建一个表示一天的时间间隔对象
one_day = datetime.timedelta(days=1)
# 创建一个表示一小时的时间间隔对象
one_hour = datetime.timedelta(hours=1)
# 创建一个表示一分钟的时间间隔对象
one_minute = datetime.timedelta(minutes=1)
# 创建一个表示一秒钟的时间间隔对象
one_second = datetime.timedelta(seconds=1)
```
你可以使用这些对象来进行日期和时间的计算,比如:
```python
import datetime
# 创建当前日期时间对象
now = datetime.datetime.now()
# 添加一天的时间间隔
tomorrow = now + datetime.timedelta(days=1)
# 添加一小时的时间间隔
next_hour = now + datetime.timedelta(hours=1)
# 添加一分钟的时间间隔
next_minute = now + datetime.timedelta(minutes=1)
# 添加一秒钟的时间间隔
next_second = now + datetime.timedelta(seconds=1)
```
以上代码演示了如何使用 `datetime.timedelta` 对象来计算日期和时间。
阅读全文
相关推荐
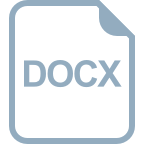
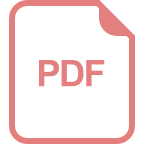
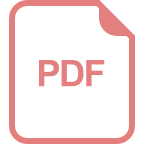
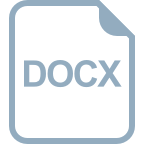
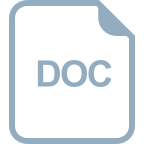











