任务:给定一个字符串,统计字符串中每个字符在该字符串中出现位置的所有下标。输出一个字典,字典的键为字符,值为该字符出现的所有下标。 举个例子:“hello” 输出结果:{"h":[0],"e":[1],"l":[2,3],"o":[4]}python
时间: 2024-09-26 17:06:28 浏览: 51
这个任务可以使用Python的内置数据结构和循环来完成。这里是一个简单的函数示例,它接受一个字符串作为输入,并返回一个字典,其中包含每个字符及其在字符串中的索引:
```python
def char_positions(string):
result = {}
for index, char in enumerate(string):
if char in result:
result[char].append(index)
else:
result[char] = [index]
return result
# 示例
example_string = "hello"
output = char_positions(example_string)
print(output) # {"h": [0], "e": [1], "l": [2, 3], "o": [4]}
```
在这个函数中,`enumerate()` 函数用于同时获取字符和它的索引。如果某个字符已经在结果字典中,我们就把当前的索引添加到对应的列表中;如果不在,就创建一个新的键值对,键是字符,值是一个只包含当前索引的列表。
相关问题
任务:给定一个字符串,统计字符串中每个字符在该字符串中出现位置的所有下标。输出一个字典,字典的键为字符,值为该字符出现的所有下标。
这是一个简单的字符串处理问题,可以使用Python来解决。你可以创建一个空字典,然后遍历输入字符串,对于每个字符,如果它已经在字典中,则将它的索引添加到对应的值列表中;如果不在字典中,就添加一个新的键值对,键是字符,值是一个只包含当前索引的一元组。
以下是Python代码示例:
```python
def char_positions(input_string):
position_dict = {}
for index, char in enumerate(input_string):
if char in position_dict:
position_dict[char].append(index)
else:
position_dict[char] = [index]
return position_dict
# 示例
input_str = "hello world"
result = char_positions(input_str)
print(result) # 输出:{'h': [0], 'e': [1], 'l': [2, 3], 'o': [4, 7], ' ': [5], 'w': [6], 'r': [8], 'd': [9]}
```
给定一个字符串,统计字符串中每个字符在该字符串中出现位置的所有下标。输出一个字典,字典的键为字符,值为该字符出现的所有下标
当您有一个字符串需要统计其中每个字符首次出现的位置,可以编写一个Python函数来完成这个任务。下面是一个示例函数:
```python
def char_positions(string):
result = {}
for index, char in enumerate(string):
if char not in result:
result[char] = [index]
else:
result[char].append(index)
return result
# 使用示例
input_string = "hello, world!"
char_dict = char_positions(input_string)
print(char_dict) # 输出: {'h': [0], 'e': [1], 'l': [2, 3], 'o': [4, 7], ', ': [5], 'w': [6], 'r': [8], 'd': [9], '!': [10]}
```
这个函数通过遍历输入字符串`string`,使用`enumerate()`获取当前字符的索引,并将其添加到对应的字符键对应的列表中,如果该字符还未出现在结果字典里则初始化一个新的列表。最后返回包含所有字符及其下标位置的字典。
阅读全文
相关推荐
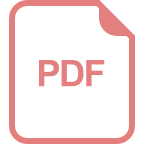
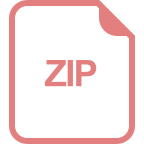
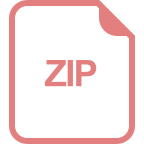

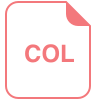








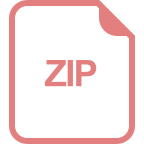
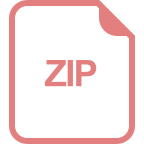
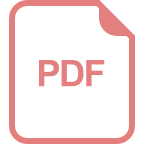