C语言给定一个单词s,统计其中各个字母出现的次数。 输入 输入一个字符串 s。 字符串s内不包含空格,s内字母均为小写。 输出 输出为出现次数不为0的字母出现多少次。 例: a出现5次,则 a=5。 样例输入 复制 str="apple"; 样例输出 复制 a=1 e=1 l=1 p=2 提示 【数据范围】 对于100%的数据,单词s长度<100000,s内字母均为小写。
时间: 2024-04-16 16:28:43 浏览: 22
你可以使用一个大小为26的数组来统计字母出现的次数,数组的下标对应字母的ASCII码减去97(即'a'的ASCII码)的值。首先将数组的所有元素初始化为0,然后遍历字符串s,每遇到一个字母就将对应的数组元素加1。遍历完成后,再遍历数组,输出出现次数不为0的字母及其出现次数。
以下是一个示例的C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s[100001];
int count[26] = {0}; // 初始化数组为0
scanf("%s", s);
int len = strlen(s);
for (int i = 0; i < len; i++) {
// 将对应字母的出现次数加1
count[s[i] - 'a']++;
}
for (int i = 0; i < 26; i++) {
if (count[i] != 0) {
// 输出出现次数不为0的字母及其出现次数
printf("%c=%d ", 'a' + i, count[i]);
}
}
return 0;
}
```
输入示例:
```
apple
```
输出示例:
```
a=1 e=1 l=1 p=2
```
相关问题
C语言给定一个字符串把其中各个单词的顺序颠倒,注意保持句首字母大写,标点符号不动。
可以使用以下步骤实现:
1. 定义一个函数,接受一个字符串参数,返回一个字符串结果。
2. 定义一个数组,存储每个单词的起始位置和长度。
3. 遍历字符串,找到每个单词的起始位置和长度,并将其存储到数组中。
4. 遍历数组,将每个单词反转。
5. 遍历数组,将每个单词的首字母大写。
6. 遍历数组,将每个单词连接起来,中间加上空格。
下面是实现该函数的代码:
```c
#include <ctype.h>
#include <string.h>
char* reverseWords(char* s) {
int len = strlen(s);
int words[100][2];
int count = 0;
int i = 0;
while (i < len) {
while (i < len && isspace(s[i])) i++;
if (i == len) break;
words[count][0] = i;
while (i < len && !isspace(s[i])) i++;
words[count][1] = i - words[count][0];
count++;
}
for (int i = 0; i < count; i++) {
int start = words[i][0];
int end = words[i][0] + words[i][1] - 1;
while (start < end) {
char tmp = s[start];
s[start] = s[end];
s[end] = tmp;
start++;
end--;
}
}
for (int i = 0; i < count; i++) {
int idx = words[i][0];
s[idx] = toupper(s[idx]);
}
char* result = malloc(len + 1);
memset(result, 0, len + 1);
for (int i = 0; i < count; i++) {
int start = words[i][0];
strncat(result, s + start, words[i][1]);
if (i < count - 1) strcat(result, " ");
}
return result;
}
```
注意,该函数返回的字符串需要手动释放。可以这样调用:
```c
char* s = "Hello, world!";
char* result = reverseWords(s);
printf("%s\n", result);
free(result);
```
C语言,给定一个字符串把其中各个单词的顺序颠倒,注意保持句首字母大写,标点符号不动。
可以使用以下步骤实现:
1. 定义一个函数,接受一个字符串参数,返回一个字符串结果。
2. 定义两个指针,分别指向字符串的开头和结尾。
3. 从结尾向开头遍历字符串,找到每个单词的结尾位置。
4. 再从每个单词的结尾位置向开头遍历,将每个单词反转。
5. 遍历字符串,将每个单词的首字母大写。
6. 遍历字符串,将每个单词连接起来,中间加上空格。
下面是实现该函数的代码:
```c
#include <ctype.h>
#include <string.h>
char* reverseWords(char* s) {
int len = strlen(s);
char* start = s;
char* end = s + len - 1;
while (isspace(*end)) end--;
char* word_end = end;
while (word_end >= start) {
if (isspace(*word_end)) {
int word_len = end - word_end;
char* word_start = word_end + 1;
for (char* p = word_start; p < end; p++) {
char tmp = *p;
*p = *word_end;
*word_end = tmp;
word_end--;
}
end = word_end;
word_end -= word_len + 1;
} else {
word_end--;
}
}
for (char* p = s; p <= end; p++) {
if (p == s || isspace(*(p - 1))) {
*p = toupper(*p);
}
}
char* result = malloc(len + 1);
memset(result, 0, len + 1);
char* p = s;
while (p <= end) {
if (!isspace(*p)) {
strncat(result, p, 1);
} else if (p > s && !isspace(*(p - 1))) {
strcat(result, " ");
}
p++;
}
return result;
}
```
注意,该函数返回的字符串需要手动释放。可以这样调用:
```c
char* s = "Hello, world!";
char* result = reverseWords(s);
printf("%s\n", result);
free(result);
```
相关推荐
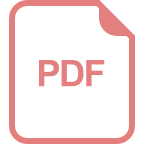
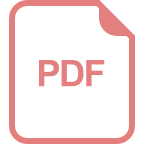












