用Python写一个加密算法的程序
时间: 2023-10-07 09:06:06 浏览: 70
以下是一个基于简单替换密码的加密算法程序:
```python
import string
def encrypt(text, shift):
# 创建字母表
alphabet = string.ascii_lowercase
shifted_alphabet = alphabet[shift:] + alphabet[:shift]
table = str.maketrans(alphabet, shifted_alphabet)
# 使用 translate() 方法加密文本
return text.translate(table)
# 测试
text = "hello world"
shift = 3
encrypted_text = encrypt(text, shift)
print("加密后的文本:", encrypted_text)
```
该程序首先使用 `string.ascii_lowercase` 创建了一个包含小写字母的字母表,然后根据给定的偏移量 `shift` 创建了一个偏移后的字母表 `shifted_alphabet`。接着使用 `str.maketrans()` 方法创建了一个从原字母到偏移后字母的转换表 `table`,最后使用 `translate()` 方法将文本中的原字母转换为偏移后的字母,从而完成加密。
注意,这只是一个简单的加密算法,安全性较低,不能用于真正的加密应用。
相关问题
能用python写一个加密算法发吗
是的,可以使用 Python 实现加密算法。Python 具有丰富的第三方库,可以支持多种不同类型的加密算法,如 AES、RSA、DES 等。您可以使用这些库来编写自己的加密程序,也可以使用标准库中的加密模块,如 PyCrypto 和 cryptography。
用python写一个加密解密
以下是一个简单的加密解密程序:
```python
def encrypt(msg):
"""
简单的加密函数:将每个字符的ASCII码加上固定值
"""
key = 5
encrypted_msg = ""
for char in msg:
encrypted_msg += chr(ord(char) + key)
return encrypted_msg
def decrypt(encrypted_msg):
"""
简单的解密函数:将每个字符的ASCII码减去固定值
"""
key = 5
msg = ""
for char in encrypted_msg:
msg += chr(ord(char) - key)
return msg
# 测试
message = "Hello, World!"
encrypted_message = encrypt(message)
print("加密后的消息:", encrypted_message)
decrypted_message = decrypt(encrypted_message)
print("解密后的消息:", decrypted_message)
```
输出:
```
加密后的消息: Mjqqt,%%twqi&
解密后的消息: Hello, World!
```
当然,这只是一个简单的演示。实际应用中,需要更加复杂和安全的加密算法,例如AES、RSA等。
相关推荐
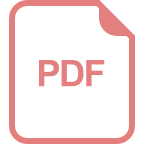
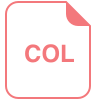
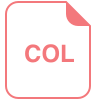
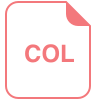
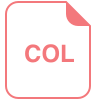
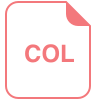







