C++ std::sort
时间: 2023-09-06 10:14:16 浏览: 64
std::sort is a function in the C++ standard library that is used to sort a range of elements in a container in ascending order. It takes two iterators as arguments, representing the beginning and end of the range to be sorted, and uses a comparison function to determine the order of the elements.
The syntax for std::sort is as follows:
```c++
template<class RandomAccessIterator>
void sort(RandomAccessIterator first, RandomAccessIterator last);
template<class RandomAccessIterator, class Compare>
void sort(RandomAccessIterator first, RandomAccessIterator last, Compare comp);
```
The first version of std::sort sorts the elements in the range [first, last) in ascending order using the default comparison operator. The second version of std::sort allows the user to provide a custom comparison function to determine the order of the elements.
std::sort uses the introsort algorithm, which is a combination of quicksort, heapsort, and insertion sort. It has an average case time complexity of O(n log n), where n is the number of elements in the range to be sorted.
Example usage:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> v {3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5};
std::sort(v.begin(), v.end());
for (auto i : v) {
std::cout << i << " ";
}
// Output: 1 1 2 3 3 4 5 5 5 6 9
}
```
相关推荐
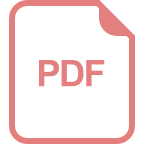
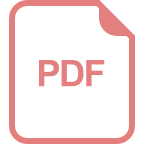
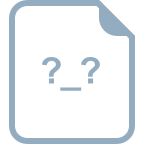














