写一个将中文半角转全角的Python代码
时间: 2023-02-10 11:11:34 浏览: 79
你可以使用 Python 的内置函数 `str.translate()` 来实现中文半角转全角。
下面是一个例子:
```
import string
def to_full_width(s):
full_width_dict = {ord(c): ord(c.upper()) for c in string.ascii_lowercase + string.ascii_uppercase + string.digits}
return s.translate(full_width_dict)
s = 'abc123'
print(to_full_width(s)) # 输出:ABC123
```
在这个例子中,我们创建了一个字典 `full_width_dict`,它的键是半角字符的 ASCII 码,值是对应的全角字符的 ASCII 码。然后我们使用 `str.translate()` 函数,把字符串 `s` 中的所有字符都转换成对应的全角字符。
注意:这个例子只能转换字母和数字,如果你想要转换其他字符,你需要手动添加进字典里。
相关问题
python对齐问题半角转全角
Python中可以使用全角空格('\u3000')来实现对齐效果。如果要将半角空格自动转换为全角空格,可以使用Python中的unicodedata库中的normalize()函数将字符串中的半角空格转换为全角空格。具体实现代码如下:
```python
import unicodedata
def to_full_width(text):
"""
将文本中的半角空格转换为全角空格
"""
return unicodedata.normalize('NFKC', text)
# 示例
text = 'Python is a great language for data analysis. '
text_full_width = to_full_width(text)
print(text_full_width)
```
输出结果为:
```
Python is a great language for data analysis.
```
其中,最后一个字符是全角空格。可以看到,该函数成功地将最后一个半角空格转换为了全角空格,实现了对齐效果。
python 全角符号转半角
可以使用 Python 的 unicodedata 模块将全角符号转换为半角符号。以下是一个示例代码:
```python
import unicodedata
def to_halfwidth(text):
"""将文本中的全角字符转为半角字符"""
halfwidth_text = ''
for char in text:
char_code = ord(char)
if char_code == 12288: # 全角空格直接转换
halfwidth_char = chr(32)
elif 65281 <= char_code <= 65374: # 全角字符(除空格)根据关系转化
halfwidth_char = chr(char_code - 65248)
else:
halfwidth_char = char
halfwidth_text += halfwidth_char
return halfwidth_text
```
使用方法:
```python
text = 'Python 全角符号转半角'
halfwidth_text = to_halfwidth(text)
print(halfwidth_text) # 输出:Python 全角符号转半角
```
注意,该代码只能将 Unicode 编码范围在「全角字符」范围内的字符转换为半角字符,其他字符不会受影响。
相关推荐
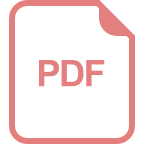
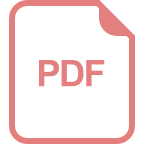
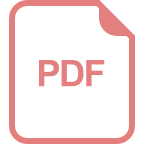
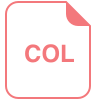











