STM32检测温度,有三个按键,第一个按键是阈值设置键,第二个按键是设置阈值加,第三个按键设置阈值减程序
时间: 2024-05-01 21:21:03 浏览: 150
可以使用STM32内部的ADC模块来检测温度,具体步骤如下:
1. 配置ADC模块,选择温度传感器作为输入源。
2. 启动ADC转换。
3. 获取ADC转换结果,转换结果即为当前温度值。
4. 根据第一个按键的状态,判断当前是否处于阈值设置模式,如果是,则根据第二个和第三个按键的状态,分别增加或减少阈值。
5. 根据当前温度值和阈值的比较结果,控制相应的输出或操作。
下面是一个简单的代码示例:
```c
#include "stm32f4xx.h"
// 定义阈值和当前温度变量
int threshold = 25;
int current_temp = 0;
int main(void)
{
// 配置ADC模块
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);
ADC_InitTypeDef ADC_InitStruct;
ADC_CommonInitTypeDef ADC_CommonInitStruct;
ADC_CommonInitStruct.ADC_Mode = ADC_Mode_Independent;
ADC_CommonInitStruct.ADC_Prescaler = ADC_Prescaler_Div2;
ADC_CommonInitStruct.ADC_DMAAccessMode = ADC_DMAAccessMode_Disabled;
ADC_CommonInitStruct.ADC_TwoSamplingDelay = ADC_TwoSamplingDelay_5Cycles;
ADC_CommonInit(&ADC_CommonInitStruct);
ADC_InitStruct.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStruct.ADC_ScanConvMode = DISABLE;
ADC_InitStruct.ADC_ContinuousConvMode = DISABLE;
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStruct.ADC_ExternalTrigConv = ADC_ExternalTrigConv_T1_CC1;
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStruct.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStruct);
ADC_Cmd(ADC1, ENABLE);
ADC_RegularChannelConfig(ADC1, ADC_Channel_TempSensor, 1, ADC_SampleTime_480Cycles);
// 配置按键GPIO口
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2;
GPIO_Init(GPIOA, &GPIO_InitStruct);
while (1)
{
// 启动ADC转换
ADC_SoftwareStartConv(ADC1);
// 等待转换完成
while (!ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC));
// 获取温度值
uint16_t adc_value = ADC_GetConversionValue(ADC1);
current_temp = (int)((1.43 - ((float)adc_value * 3.3 / 4096)) / 0.0043 + 25);
// 检测按键状态
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == RESET)
{
// 进入阈值设置模式
while (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == RESET)
{
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_1) == RESET)
{
// 增加阈值
threshold++;
}
else if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_2) == RESET)
{
// 减少阈值
threshold--;
}
}
}
// 控制输出或操作
if (current_temp > threshold)
{
// 温度高于阈值,执行某些操作
}
else
{
// 温度低于阈值,执行某些操作
}
}
}
```
阅读全文
相关推荐
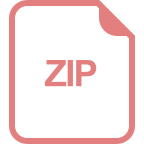
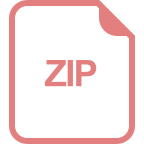
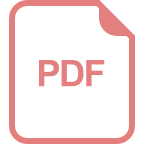
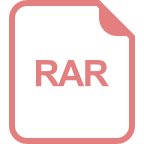
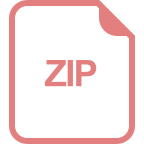
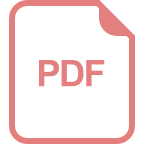
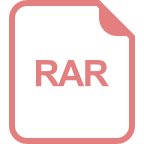
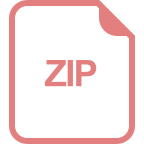
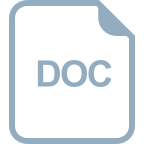
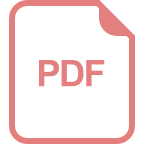
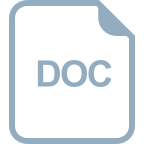
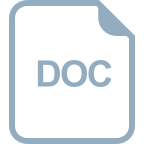
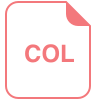
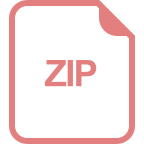