如果需要读取指定的关键字呢
时间: 2023-12-03 13:05:43 浏览: 120
如果需要在 TTC 字体文件中读取指定的关键字,可以通过以下步骤实现:
1. 使用 `font-encoder` 依赖读取 TTC 字体文件。
2. 获取字体中的所有字符映射表(cmap)。
```java
TrueTypeFont ttf = TrueTypeFont.createFont(inputStream);
CMapTable cmapTable = ttf.getTableMap().getCMapTable();
```
3. 根据关键字获取字符编码。
可以通过 `getCMaps()` 方法获取所有的字符映射表,然后遍历所有的字符映射表,查找包含关键字的字符编码。例如,查找包含关键字 `hello` 的字符编码:
```java
int[] codes = null;
for (CMap cmap : cmapTable.getCMaps()) {
if (cmap.getPlatformId() == 3 && cmap.getPlatformEncodingId() == 1) {
codes = cmap.getGlyphIds("hello");
if (codes != null && codes.length > 0) {
break;
}
}
}
```
其中,`getPlatformId()` 和 `getPlatformEncodingId()` 方法用于指定字符映射表的平台 ID 和平台编码 ID,这里使用的是 Windows 平台的 UTF-16 编码。
4. 根据字符编码创建字体。
```java
Font font = ttf.getFont().deriveFont(16f);
```
5. 使用字体绘制文本。
```java
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = image.createGraphics();
g2d.setFont(font);
g2d.drawString("hello", x, y);
g2d.dispose();
```
例如,使用 `font-encoder` 依赖在 TTC 字体文件中查找包含关键字 `hello` 的字符编码:
```java
import java.awt.Font;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.FileInputStream;
import java.io.InputStream;
import com.github.harukawa.ttfparser.CMap;
import com.github.harukawa.ttfparser.CMapTable;
import com.github.harukawa.ttfparser.TrueTypeFont;
// 读取字体文件
InputStream inputStream = new FileInputStream("font.ttc");
TrueTypeFont ttf = TrueTypeFont.createFont(inputStream);
// 查找字符编码
int[] codes = null;
CMapTable cmapTable = ttf.getTableMap().getCMapTable();
for (CMap cmap : cmapTable.getCMaps()) {
if (cmap.getPlatformId() == 3 && cmap.getPlatformEncodingId() == 1) {
codes = cmap.getGlyphIds("hello");
if (codes != null && codes.length > 0) {
break;
}
}
}
// 创建字体
Font font = ttf.getFont().deriveFont(16f);
// 绘制文本
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = image.createGraphics();
g2d.setFont(font);
g2d.drawString("hello", x, y);
g2d.dispose();
```
阅读全文
相关推荐
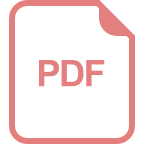
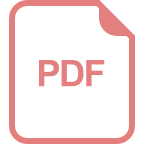
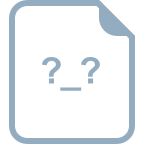
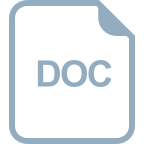











