怎么用itextpdf读取ttc库中指定的关键字到新的字体文件中
时间: 2024-02-18 16:58:58 浏览: 258
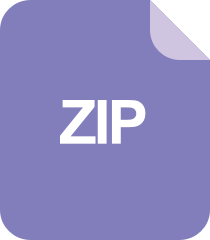
iText生成pdf解决中文不显示
要使用 iTextPDF 读取 TTC 字体库中指定的关键字并将其复制到新的字体文件中,可以使用以下步骤:
1. 创建一个 `PdfFont` 对象,指定 TTC 字体文件和字体索引,以及字体大小、样式等属性。
```
String fontPath = "path/to/font.ttc";
int fontIndex = 0; // the index of the font in the TTC file
float fontSize = 12;
int fontStyle = Font.NORMAL;
PdfFont font = PdfFontFactory.createFont(fontPath + "," + fontIndex, PdfEncodings.IDENTITY_H, true);
PdfFont newFont = PdfFontFactory.createFont();
newFont.setPdfFontName(font.getPdfFontName());
newFont.setSubset(true);
newFont.setBold(fontStyle == Font.BOLD);
newFont.setItalic(fontStyle == Font.ITALIC);
```
2. 创建一个 `PdfDocument` 对象,用于读取和写入 PDF 文件。
```
PdfDocument pdfDoc = new PdfDocument(new PdfReader("path/to/input.pdf"), new PdfWriter("path/to/output.pdf"));
```
3. 遍历 PDF 文件中的所有页面,查找指定的关键字,并将其复制到新的字体文件中。
```
for (int i = 1; i <= pdfDoc.getNumberOfPages(); i++) {
PdfPage page = pdfDoc.getPage(i);
PdfDictionary pageDict = page.getPdfObject();
PdfArray annotsArray = pageDict.getAsArray(PdfName.Annots);
if (annotsArray == null) {
continue;
}
for (int j = 0; j < annotsArray.size(); j++) {
PdfDictionary annotDict = annotsArray.getAsDictionary(j);
if (annotDict == null) {
continue;
}
PdfString content = annotDict.getAsString(PdfName.Contents);
if (content != null && content.getValue().contains("关键字")) {
String newContent = content.getValue().replace("关键字", "");
PdfString newString = new PdfString(newContent, PdfEncodings.IDENTITY_H);
annotDict.put(PdfName.Contents, newString);
}
PdfArray apDict = annotDict.getAsArray(PdfName.AP);
if (apDict != null) {
PdfDictionary normalDict = apDict.getAsDictionary(0);
PdfStream stream = normalDict.getAsStream(PdfName.Stream);
byte[] bytes = stream.getBytes();
String str = new String(bytes, PdfEncodings.IDENTITY_H);
String newStr = str.replace("关键字", "");
stream.setData(newStr.getBytes(PdfEncodings.IDENTITY_H));
}
}
}
```
4. 关闭 `PdfDocument` 对象。
```
pdfDoc.close();
```
在上面的示例代码中,我们首先创建了一个 `PdfFont` 对象,指定 TTC 字体文件和字体索引,以及字体大小、样式等属性。然后,我们创建了一个 `PdfDocument` 对象,用于读取和写入 PDF 文件。接下来,我们遍历 PDF 文件中的所有页面,查找指定的关键字,并将其复制到新的字体文件中。最后,我们关闭了 `PdfDocument` 对象。
请注意,上面的示例代码仅演示了如何读取 PDF 文件中的注释和文本字段,并替换其中的关键字。如果您需要读取 PDF 文件中的其他内容,例如文本、表格或图像等,请使用适当的 iTextPDF 类库和方法进行处理。
阅读全文
相关推荐
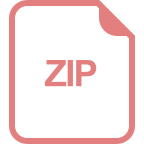
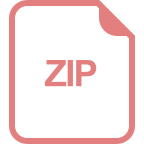







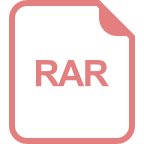
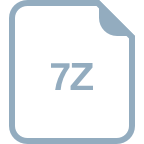






