帮我用c++写飞机订票管理系统
时间: 2023-07-19 15:11:44 浏览: 169
好的,下面是一个简单的飞机订票管理系统的C++代码:
```c++
#include <iostream>
#include <string>
using namespace std;
const int ROWS = 10;
const int COLS = 6;
class Flight {
private:
string flightNo;
string destination;
int seatMap[ROWS][COLS];
int availableSeats;
public:
Flight(string flightNo, string destination) {
this->flightNo = flightNo;
this->destination = destination;
this->availableSeats = ROWS * COLS;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
seatMap[i][j] = 0;
}
}
}
void displaySeatMap() {
cout << "Seat Map for Flight " << flightNo << endl;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cout << seatMap[i][j] << " ";
}
cout << endl;
}
}
bool bookSeat(int row, int col) {
if (seatMap[row-1][col-1] == 0) {
seatMap[row-1][col-1] = 1;
availableSeats--;
return true;
}
else {
return false;
}
}
void displayFlightDetails() {
cout << "Flight No: " << flightNo << endl;
cout << "Destination: " << destination << endl;
cout << "Available Seats: " << availableSeats << endl;
}
};
int main() {
Flight f1("123", "New York");
f1.displaySeatMap();
f1.bookSeat(1, 1);
f1.bookSeat(2, 3);
f1.bookSeat(5, 4);
f1.displaySeatMap();
f1.displayFlightDetails();
return 0;
}
```
这个程序定义了一个Flight类,用于表示一个航班,包括航班号,目的地,座位图和可用座位数量等信息。程序还提供了一些方法,用于显示座位图,预订座位和显示航班详情等功能。在main函数中,我们创建了一个Flight对象f1,并调用了一些方法来测试该类的功能。
阅读全文
相关推荐
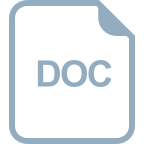
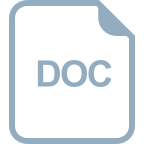
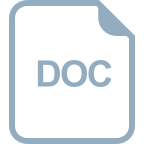
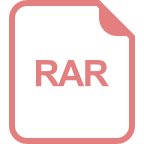
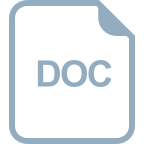
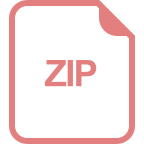
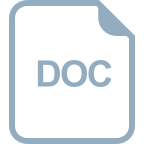
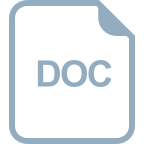
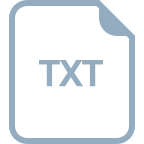
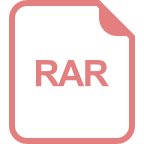
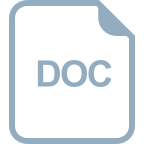
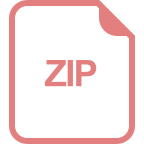
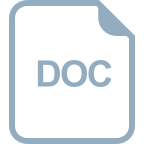
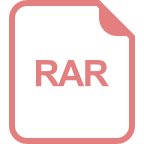
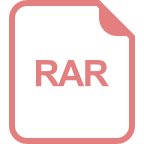
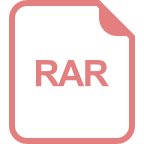
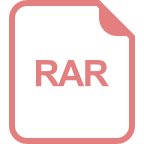

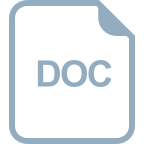