用c++写一个简易的飞机订票管理系统
时间: 2023-11-07 20:04:45 浏览: 131
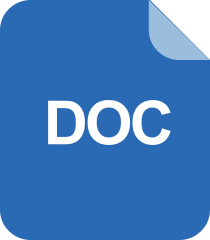
C++飞机订票管理系统.doc

好的,下面是一个简单的飞机订票管理系统的C++代码:
```cpp
#include<iostream>
#include<string>
using namespace std;
class Flight {
public:
string flightNumber;
string departureCity;
string arrivalCity;
int availableSeatNum;
int *seatStatus;
Flight(string fn, string dc, string ac, int asn) {
flightNumber = fn;
departureCity = dc;
arrivalCity = ac;
availableSeatNum = asn;
seatStatus = new int[asn];
for (int i = 0; i < asn; i++) {
seatStatus[i] = 0;
}
}
~Flight() {
delete[] seatStatus;
}
void displayFlightInfo() {
cout << "Flight Number: " << flightNumber << endl;
cout << "Departure City: " << departureCity << endl;
cout << "Arrival City: " << arrivalCity << endl;
cout << "Available Seat Number: " << availableSeatNum << endl;
}
bool isSeatAvailable(int seatNum) {
if (seatNum < 1 || seatNum > availableSeatNum) {
return false;
}
if (seatStatus[seatNum - 1] == 1) {
return false;
}
return true;
}
bool bookSeat(int seatNum) {
if (isSeatAvailable(seatNum)) {
seatStatus[seatNum - 1] = 1;
availableSeatNum--;
return true;
}
return false;
}
void displaySeatStatus() {
cout << "Seat Status:" << endl;
for (int i = 0; i < availableSeatNum; i++) {
cout << "Seat " << i + 1 << ": ";
if (seatStatus[i] == 0) {
cout << "Available" << endl;
}
else {
cout << "Booked" << endl;
}
}
}
};
class BookingSystem {
public:
Flight **flights;
int numFlights;
BookingSystem() {
flights = NULL;
numFlights = 0;
}
~BookingSystem() {
if (flights != NULL) {
for (int i = 0; i < numFlights; i++) {
delete flights[i];
}
delete[] flights;
}
}
void addFlight(string fn, string dc, string ac, int asn) {
Flight **newFlights = new Flight*[numFlights + 1];
for (int i = 0; i < numFlights; i++) {
newFlights[i] = flights[i];
}
newFlights[numFlights] = new Flight(fn, dc, ac, asn);
numFlights++;
if (flights != NULL) {
delete[] flights;
}
flights = newFlights;
}
void displayAllFlightsInfo() {
for (int i = 0; i < numFlights; i++) {
flights[i]->displayFlightInfo();
}
}
Flight* findFlight(string fn) {
for (int i = 0; i < numFlights; i++) {
if (flights[i]->flightNumber == fn) {
return flights[i];
}
}
return NULL;
}
bool bookSeat(string fn, int seatNum) {
Flight *flight = findFlight(fn);
if (flight == NULL) {
return false;
}
return flight->bookSeat(seatNum);
}
void displaySeatStatus(string fn) {
Flight *flight = findFlight(fn);
if (flight != NULL) {
flight->displaySeatStatus();
}
}
};
int main() {
BookingSystem bookingSystem;
bookingSystem.addFlight("CA123", "Shanghai", "Beijing", 100);
bookingSystem.addFlight("CA456", "Beijing", "Shanghai", 80);
int choice;
while (true) {
cout << "1. Display all flights information" << endl;
cout << "2. Book a seat" << endl;
cout << "3. Display seat status" << endl;
cout << "0. Exit" << endl;
cout << "Please input your choice: ";
cin >> choice;
if (choice == 0) {
break;
}
else if (choice == 1) {
bookingSystem.displayAllFlightsInfo();
}
else if (choice == 2) {
string fn;
int seatNum;
cout << "Please input flight number: ";
cin >> fn;
cout << "Please input seat number: ";
cin >> seatNum;
if (bookingSystem.bookSeat(fn, seatNum)) {
cout << "Booked successfully!" << endl;
}
else {
cout << "Booking failed!" << endl;
}
}
else if (choice == 3) {
string fn;
cout << "Please input flight number: ";
cin >> fn;
bookingSystem.displaySeatStatus(fn);
}
else {
cout << "Invalid input, please try again!" << endl;
}
}
return 0;
}
```
该程序使用了面向对象的设计思想,定义了两个类:Flight和BookingSystem。Flight类表示航班,包含了航班号、出发城市、到达城市、可用座位数和座位状态等属性,以及一些查询航班信息、查询座位状态、预订座位等方法。BookingSystem类表示订票系统,包含了多个航班,以及一些添加航班、查询航班、预订座位等方法。
在主函数中,用户可以选择查看所有航班信息、预订座位、查询座位状态等操作。当用户选择预订座位时,程序会要求用户输入航班号和座位号,并根据输入的信息预订座位。当用户选择查询座位状态时,程序会要求用户输入航班号,并显示该航班的座位状态。
阅读全文
相关推荐
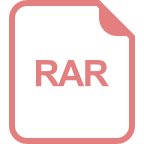
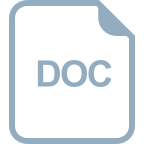
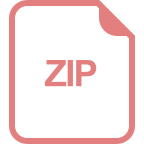
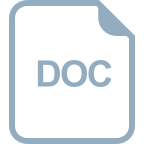
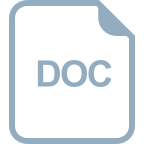
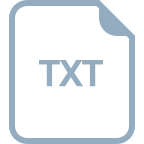
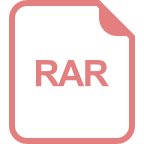
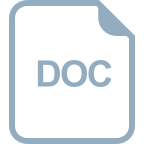



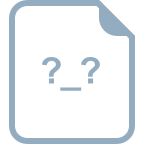
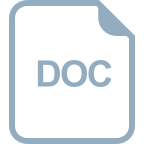
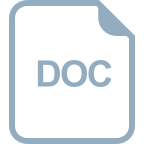