利用PIL库显示图像以及相应的R、G、B三个彩色图像。
时间: 2024-12-19 15:27:34 浏览: 16
要利用PIL库显示图像以及提取并展示其R、G、B三个彩色通道,你可以按照以下步骤操作:
首先,确保已安装PIL库,如果尚未安装,可以使用`pip install pillow`命令安装[^1]。
1. **加载图像**
```python
from PIL import Image
# 加载一张图像,这里以.jpg为例
img_path = "path_to_your_image.jpg"
image = Image.open(img_path)
```
2. **查看原始图像**
```python
image.show() # 显示原图像
```
3. **分离R、G、B通道**
使用`.convert('RGB')`将图像从可能的其他模式转换为RGB模式,然后通过`.split()`方法分离每个通道
```python
r, g, b = image.convert('RGB').split()
```
4. **显示单通道图像**
```python
r.show(title="Red Channel") # 展示红色通道
g.show(title="Green Channel") # 展示绿色通道
b.show(title="Blue Channel") # 展示蓝色通道
```
这样就可以看到图像的每个单独的颜色通道了。
相关问题
利用PIL库显示红绿蓝三个通道彩色图片
要利用PIL库显示红绿蓝(RGB)三个通道的彩色图片,你可以先加载一个支持RGB模式的图片,然后对每个通道进行独立操作并合并。这里是简单的示例:
```python
from PIL import Image
# 加载支持RGB模式的图片
def display_RGB_channels(image_path):
# 读取图像
img = Image.open(image_path)
# 检查图像是否是RGB模式,如果不是,可以使用convert('RGB')转换
if img.mode != 'RGB':
img = img.convert('RGB')
# 提取每个通道
r_channel, g_channel, b_channel = img.split()
# 对每个通道进行展示,这里只是简单地打印出来,实际可以保存为单独的图片
print(f"Red channel:")
display_img(r_channel)
print(f"Green channel:")
display_img(g_channel)
print(f"Blue channel:")
display_img(b_channel)
# 合并通道并显示原图
original_img = Image.merge('RGB', (r_channel, g_channel, b_channel))
display_img(original_img)
def display_img(channel):
# 创建一个新的空白图像用于显示单个通道
img_to_show = Image.new('RGB', (channel.width, channel.height), (255, 255, 255))
draw = ImageDraw.Draw(img_to_show)
for y in range(channel.height):
for x in range(channel.width):
pixel = channel.getpixel((x, y))
draw.point((x, y), tuple(pixel))
img_to_show.show()
# 使用示例
image_path = "your_image_path.jpg"
display_RGB_channels(image_path)
```
在这个示例中,`display_RGB_channels`函数会打开指定路径的图片,提取RGB三个通道,分别显示每个通道的内容,并最后合并回原始图像。
b)提取彩色图像的RGB三个波段(Parrots_R, Parrots_G和Parrots_B),利用subplot()函数在一个figure中同时显示彩色图像以及相应R、G和B三个波段灰度图像(利用subplot(2,2,i)显示);
在Python的Matplotlib库中,你可以使用`PIL`(Pillow)库读取彩色图像,然后通过`numpy`处理每个颜色通道(RGB)。以下是一个示例步骤:
首先,确保已安装Pillow库,如果没有可以使用pip安装:
```bash
pip install pillow
```
然后,你可以按照以下代码来实现:
```python
import matplotlib.pyplot as plt
from PIL import Image
# 加载彩色图像
img = Image.open('parrots.jpg') # 请替换为你实际的图片路径
rgb_array = np.array(img)
# 提取RGB三个波段
Parrots_R = rgb_array[:, :, 0] # 第0通道对应红色
Parrots_G = rgb_array[:, :, 1] # 第1通道对应绿色
Parrots_B = rgb_array[:, :, 2] # 第2通道对应蓝色
# 创建一个新的figure
plt.figure(figsize=(8, 4))
# 使用subplot显示彩色图像和RGB灰度图
for i in range(1, 5):
if i <= 3:
ax = plt.subplot(2, 2, i)
ax.imshow(rgb_array, cmap='gray') # 显示彩色图像转成的灰度图像
ax.set_title(f"Color Channel {i}")
else:
ax.imshow(Parrots_R, cmap='gray', title="Red") if i == 4 else \
ax.imshow(Parrots_G, cmap='gray', title="Green") if i == 5 else \
ax.imshow(Parrots_B, cmap='gray', title="Blue")
# 显示图像
plt.tight_layout()
plt.show()
```
这里,我们创建了一个2x2的网格布局(subplot),前两个子图展示的是彩色图像的灰度版本,后三个分别显示了单独的红、绿、蓝三个通道。
阅读全文
相关推荐
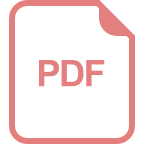
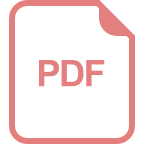
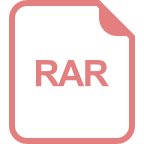
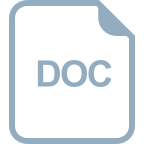
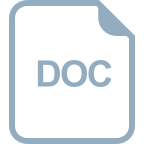
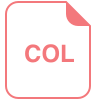










