easyx如何实现图像旋转
时间: 2024-06-21 10:03:02 浏览: 437
EasyX是一个轻量级的C++图形库,它提供了一套简单易用的接口来处理图像旋转。在EasyX中,图像旋转通常是通过矩阵变换来实现的,特别是使用仿射变换。以下是实现图像旋转的基本步骤:
1. **创建旋转矩阵**:
使用旋转变换公式创建一个二维旋转矩阵,该矩阵描述了旋转的角度和原点。对于顺时针旋转,角度为正;逆时针旋转则为负。原点通常设为图像的左上角。
```cpp
float angle_radians = degrees_to_radians(rotation_angle);
float cos_theta = cos(angle_radians);
float sin_theta = sin(angle_radians);
// 如果顺时针旋转,将sin_theta和cos_theta取相反数
if (rotation_angle < 0) {
cos_theta = -cos_theta;
sin_theta = -sin_theta;
}
float matrix = {
{ cos_theta, -sin_theta, x_offset, 0 },
{ sin_theta, cos_theta, y_offset, 0 },
{ 0, 0, 1, 0 },
{ 0, 0, 0, 1 }
};
```
2. **应用矩阵变换**:
将图像的每个像素坐标(x, y)乘以旋转矩阵,得到新的坐标(x_prime, y_prime),从而实现旋转。
```cpp
for (int y = 0; y < image_height; ++y) {
for (int x = 0; x < image_width; ++x) {
int newX = round(matrix*x + matrix*y + matrix);
int newY = round(matrix*x + matrix*y + matrix);
// 将新的坐标映射到旋转后的图像区域
newX = std::min(std::max(0, newX), width - 1);
newY = std::min(std::max(0, newY), height - 1);
// 读取原像素并写入新位置
rotated_image[newY][newX] = image[y][x];
}
}
```
3. **可能的优化**:
由于旋转操作可能会导致像素重叠或丢失,实际应用中可能会使用像素插值(如Bilinear、Nearest等)来平滑地从周围的像素取值。
**相关问题--:**
1. EasyX库是否支持其他类型的图像变换?
2. 有没有内置的方法可以直接进行图像旋转,而无需手动计算矩阵?
3. 图像旋转对性能有什么影响,如何提高旋转效率?
阅读全文
相关推荐
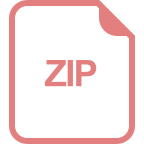
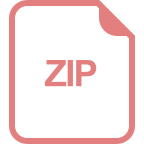
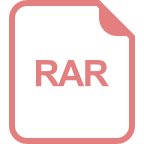















