展示一段NSGA-Ⅱ的交叉操作的python代码,逐行解释代码的含义
时间: 2024-12-23 09:24:30 浏览: 6
NSGA-II (Non-dominated Sorting Genetic Algorithm II) 是一种用于多目标优化问题的进化算法。下面是一个简单的Python代码片段,展示的是NSGA-II中的单点交叉操作:
```python
import numpy as np
def single_point_crossover(parents, crossover_rate):
# 获取交叉率
num_individuals = len(parents)
crossover_mask = np.random.rand(num_individuals) < crossover_rate
offspring = []
for i in range(0, num_individuals, 2): # 因为每次处理一对父母
if crossover_mask[i]: # 如果需要交叉
point = np.random.randint(0, parents[0].shape[0]) # 选择交叉点
child1 = np.concatenate((parents[i][:point], parents[i+1][point:]), axis=0)
child2 = np.concatenate((parents[i+1][:point], parents[i][point:]), axis=0)
else:
child1 = parents[i]
child2 = parents[i+1]
offspring.extend([child1, child2])
return offspring
```
逐行解释:
1. `import numpy as np`:导入NumPy库,用于处理数组操作。
2. `crossover_rate` 定义了交叉操作的发生概率。
3. `num_individuals = len(parents)`:计算父母群体的数量。
4. `crossover_mask = np.random.rand(num_individuals) < crossover_rate`:生成随机数矩阵,每个位置的概率等于交叉率,大于这个值的位置设置为True,表示进行交叉。
5. `offspring = []`:初始化结果子代列表。
6. `for i in range(0, num_individuals, 2)`: 进行两两配对,因为每次循环处理一对父母。
7-8. `if crossover_mask[i]`: 判断是否进行交叉。如果True,继续进行交叉操作。
9-11. `point = np.random.randint(0, parents[0].shape[0])`: 随机选择交叉点。
12-14. `child1 = np.concatenate((parents[i][:point], parents[i+1][point:])`, `child2 = np.concatenate((parents[i+1][:point], parents[i][point:])`):通过交叉点分割父代,然后拼接形成子代。
15-17. `else:` 如果不进行交叉,则直接复制父代个体。
18. `offspring.extend([child1, child2])`:将新产生的子代添加到结果列表中。
阅读全文
相关推荐
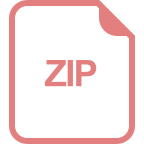
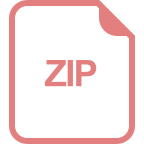
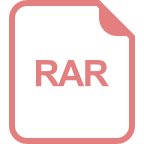

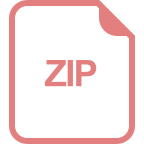
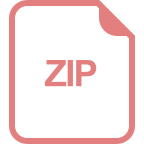
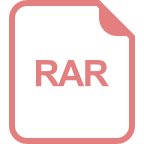
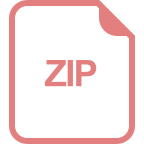









