如何使用C++实现一个基于单链表的学生信息管理系统,并包含基本的增删查改功能?
时间: 2024-11-19 15:38:01 浏览: 0
要实现一个基于单链表的学生信息管理系统,并包含基本的增删查改功能,你可以参考《C++实现学生信息管理系统单链表操作及源代码分析》这份资料。在这份资料中,你将找到关于如何定义单链表节点、初始化链表、添加和删除节点、按条件查找节点以及输出链表内容的详细解释和示例代码。以下是一个简单而全面的指南,帮助你入门和掌握这些关键操作:
参考资源链接:[C++实现学生信息管理系统单链表操作及源代码分析](https://wenku.csdn.net/doc/8bwnopsdir?spm=1055.2569.3001.10343)
1. **定义单链表节点和初始化链表**:首先定义学生信息的数据结构,然后实现一个函数`InitList`来创建一个空的链表。
```cpp
struct StudentNode {
int id;
string name;
float score;
StudentNode* next;
};
void InitList(StudentNode*& head) {
head = new StudentNode();
head->next = nullptr;
}
```
2. **添加学生信息节点**:实现`ListInsert`函数在链表中插入新的学生信息节点。
```cpp
bool ListInsert(StudentNode*& head, int pos, StudentNode* newNode) {
if (pos < 0 || pos > ListLength(head)) return false;
StudentNode* current = head;
int index = 0;
while (current != nullptr && index < pos - 1) {
current = current->next;
index++;
}
newNode->next = current->next;
current->next = newNode;
return true;
}
```
3. **删除学生信息节点**:通过`ListDelete`函数删除链表中的特定节点。
```cpp
bool ListDelete(StudentNode*& head, int pos) {
if (pos < 0 || pos >= ListLength(head)) return false;
StudentNode* current = head;
int index = 0;
while (current->next != nullptr && index < pos) {
current = current->next;
index++;
}
if (current->next == nullptr) return false;
StudentNode* toDelete = current->next;
current->next = toDelete->next;
delete toDelete;
return true;
}
```
4. **查找学生信息**:实现`Search_no`和`Search_name`函数分别通过学号和名字查找学生信息。
```cpp
StudentNode* Search_no(StudentNode* head, int no) {
StudentNode* current = head->next;
while (current != nullptr) {
if (current->id == no) return current;
current = current->next;
}
return nullptr;
}
StudentNode* Search_name(StudentNode* head, string name) {
StudentNode* current = head->next;
while (current != nullptr) {
if (current->name == name) return current;
current = current->next;
}
return nullptr;
}
```
5. **输出学生信息**:通过`printlist_Link`函数输出链表中所有学生的信息。
```cpp
void printlist_Link(StudentNode* head) {
StudentNode* current = head->next;
while (current != nullptr) {
cout <<
参考资源链接:[C++实现学生信息管理系统单链表操作及源代码分析](https://wenku.csdn.net/doc/8bwnopsdir?spm=1055.2569.3001.10343)
阅读全文
相关推荐
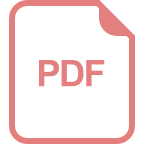
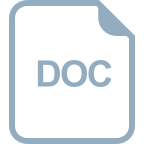
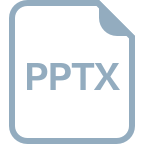


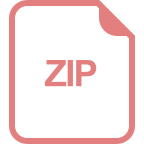
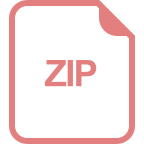
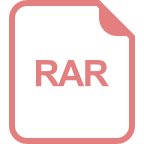
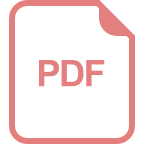
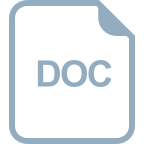
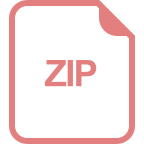
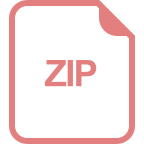
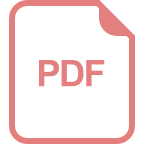
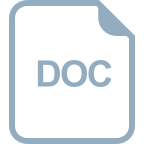
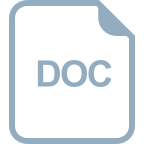
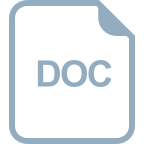
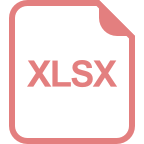
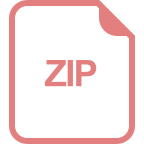
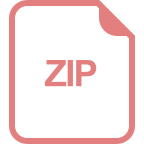
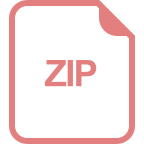