用c 语言完成利用线性表存储及相关操作,实现班上同学通讯录的管理(增、改、插、查等功能)。
时间: 2024-10-20 22:03:58 浏览: 31
在C语言中,我们可以使用结构体和指针来创建一个简单的线性表数据结构,用于模拟班级同学通讯录的功能。首先,定义一个结构体`Student`,包含姓名`name`和电话号码`phone`:
```c
struct Student {
char name[50];
char phone[20];
};
```
接下来,我们定义一个动态数组(这里用链表表示线性表),并实现基本的操作:
1. **添加学生**(插入):
```c
void add_student(Student *list, int index, const char *name, const char *phone) {
if (index < 0 || index > list_size) {
printf("Invalid index.\n");
return;
}
struct Student new_student = {name, phone};
list[index] = new_student;
}
```
2. **修改学生信息**(更新):
```c
void update_student(Student **list, int index, const char *name, const char *phone) {
if (index < 0 || index >= list_size) {
printf("Invalid index.\n");
return;
}
list[index].name = name;
list[index].phone = phone;
}
```
3. **查找学生**(搜索):
```c
void search_student(const Student *list, int index, const char *name) {
if (index < 0 || index >= list_size) {
printf("Student not found.\n");
return;
}
if(strcmp(list[index].name, name) == 0) {
printf("Name: %s, Phone: %s\n", list[index].name, list[index].phone);
} else {
printf("Student not found with the given name.\n");
}
}
```
4. **删除学生**(移除):
```c
void delete_student(Student **list, int index) {
if (index < 0 || index >= list_size) {
printf("Invalid index.\n");
return;
}
memmove(&(*list)[index], &(*list)[index + 1], sizeof(struct Student) * (list_size - index - 1));
list_size--;
}
```
注意,这里的`list_size`需要维护,初始化时设为0,每次操作后根据实际情况调整。
阅读全文
相关推荐
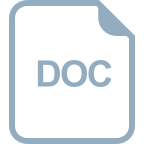
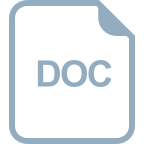
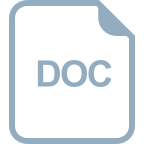

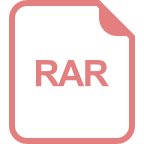
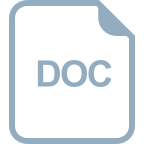
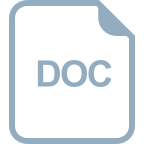
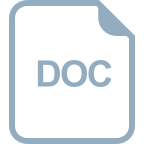





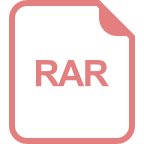
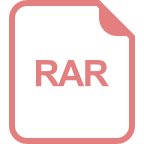


