用C++ 复制一份copy的文件并重新命名为new。只能用open read wite close函数
时间: 2024-09-09 22:11:41 浏览: 92
在C++中,你可以使用`fstream`库来复制文件并将其重命名。这里是一个简单的示例,展示了如何使用`ifstream`读取源文件,`ofstream`写入目标文件,并在完成后关闭它们:
```cpp
#include <fstream>
#include <string>
void copyFile(const std::string& srcFileName, const std::string& newFileName) {
// 打开源文件和目标文件
ifstream in(srcFileName, ios::binary);
ofstream out(newFileName, ios::binary);
if (!in || !out) {
// 检查是否成功打开,如果失败则输出错误信息并退出
std::cerr << "Failed to open file!" << std::endl;
return;
}
// 读取源文件内容到缓冲区
char buffer[4096];
while (in.read(buffer, sizeof(buffer))) {
// 写入缓冲区的内容到目标文件
out.write(buffer, in.gcount());
}
// 如果还有剩余未读取的数据,处理它
if (in.gcount() != 0) {
in.clear(); // 清除输入流中的错误标志
in.seekg(0, std::ios_base::end); // 移动到文件末尾
out.seekp(out.tellp() - in.gcount(), std::ios_base::cur); // 向后移动已读数据的位置
in.read(buffer, in.gcount()); // 读取余下的部分
out.write(buffer, in.gcount()); // 写入目标文件
}
// 关闭文件
in.close();
out.close();
if (!in.is_open() || !out.is_open()) {
std::cerr << "Error closing files." << std::endl;
} else {
std::cout << "File copied successfully and renamed to " << newFileName << "." << std::endl;
}
}
// 调用函数
int main() {
copyFile("oldfile.txt", "newfile.txt");
return 0;
}
```
阅读全文
相关推荐
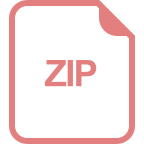
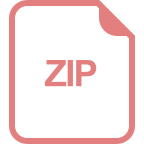
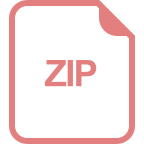
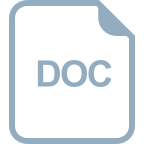











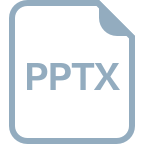
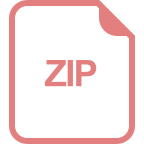