复制一份copy文件并重新命名为new。只能用open read wite close函数
时间: 2024-09-09 09:11:15 浏览: 57
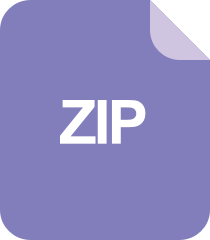
pargser:ruby gem to wite CLI keysargs 以 ruby 方式解析(例如简单且很快)
在编程中,复制文件并将其重命名为新文件名通常涉及以下步骤:
1. 使用`open`函数打开原始文件。这个函数需要两个参数:文件名和模式。对于读取文件,应该使用模式`'r'`。
2. 创建一个新文件名,并使用`open`函数以写入模式打开新文件。模式应该是`'w'`,这将允许写入新内容到文件中。
3. 使用`read`函数读取原始文件的全部内容,并将读取的数据存储到一个变量中。
4. 使用`write`函数将读取的数据写入到新文件中。
5. 关闭原始文件和新文件。使用`close`函数确保所有数据都被正确地写入磁盘,并释放系统资源。
以下是这个过程的一个简单伪代码表示:
```python
# 打开原始文件
original_file = open('copy', 'r')
# 打开新文件准备写入
new_file = open('new', 'w')
# 读取原始文件内容
file_content = original_file.read()
# 将内容写入新文件
new_file.write(file_content)
# 关闭文件
original_file.close()
new_file.close()
```
请注意,这段伪代码假设文件`copy`存在,并且你有权限读取和写入文件。
阅读全文
相关推荐
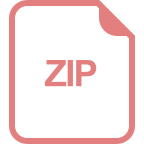
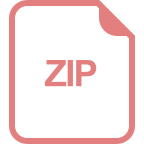


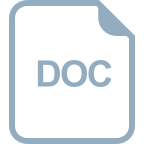








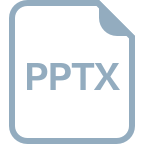
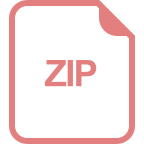