抽象一个能表示水平等腰梯形的Trapezoid类,再设计一个测试类,联合其他形状类一起测试
时间: 2024-10-12 15:08:01 浏览: 12
首先,我们来抽象出一个名为`Trapezoid`的类,它代表一个水平等腰梯形。这个类通常会包含属性如上底、下底、高以及两个等高的角度(因为等腰梯形的两侧角度相等)。我们可以这样设计:
```java
public abstract class Trapezoid {
private double topBase;
private double bottomBase;
private double height;
private double angle;
public Trapezoid(double topBase, double bottomBase, double height, double angle) {
this.topBase = topBase;
this.bottomBase = bottomBase;
this.height = height;
this.angle = Math.toRadians(angle);
}
// 公共方法
public double getTopBase() { return topBase; }
public double getBottomBase() { return bottomBase; }
public double getHeight() { return height; }
public double getAngle() { return angle; }
// 抽象方法,用于计算面积(需要子类实现)
public abstract double calculateArea();
// 计算周长,这里可以通用
public double calculatePerimeter() {
return topBase + bottomBase + 2 * Math.tan(angle) * height;
}
}
```
然后,为了创建一个具体的等腰梯形子类,比如`IsoscelesTrapezoid`,我们需要重写`calculateArea()`方法:
```java
public class IsoscelesTrapezoid extends Trapezoid {
public IsoscelesTrapezoid(double topBase, double bottomBase, double height) {
super(topBase, bottomBase, height, Math.PI / 4); // 假设角度是45度,如果是等腰则角度固定
}
@Override
public double calculateArea() {
return (topBase + bottomBase) * height / 2;
}
}
```
接着,我们可以设计一个测试类`ShapeTest`来测试我们的`Trapezoid`和`IsoscelesTrapezoid`:
```java
public class ShapeTest {
public static void main(String[] args) {
Trapezoid trapezoid = new IsoscelesTrapezoid(5, 8, 4);
System.out.println("Trapezoid with top base " + trapezoid.getTopBase() +
", bottom base " + trapezoid.getBottomBase() +
", and height " + trapezoid.getHeight() + ":");
System.out.println("Area: " + trapezoid.calculateArea());
System.out.println("Perimeter: " + trapezoid.calculatePerimeter());
// 测试其他形状类,例如Circle, Rectangle等(此处省略)
}
}
```
阅读全文
相关推荐
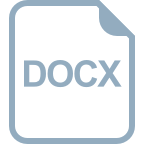
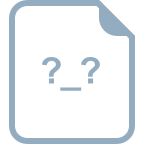
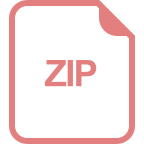













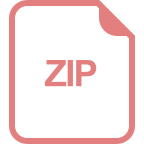