定义一个抽象类Shape,该类有抽象方法area,圆形、矩形、梯形等均是一种Shape,也都有自己求area方法,定义这些类并写测试类进行测试。
时间: 2024-12-17 14:51:02 浏览: 6
首先,我们可以定义一个抽象类`Shape`,它有一个抽象方法`area`,表示所有形状都有面积计算的基本特性:
```java
// 抽象Shape类
abstract class Shape {
abstract double area();
}
```
然后分别定义具体的形状类,如`Circle`, `Rectangle`, 和 `Trapezoid`:
```java
// Circle类
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
double area() {
return Math.PI * radius * radius;
}
}
// Rectangle类
class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
double area() {
return width * height;
}
}
// Trapezoid类 (这里简化处理,假设直角梯形)
class Trapezoid extends Shape {
private double base1;
private double base2;
private double height;
public Trapezoid(double base1, double base2, double height) {
this.base1 = base1;
this.base2 = base2;
this.height = height;
}
@Override
double area() {
return 0.5 * (base1 + base2) * height;
}
}
```
接下来,我们可以编写一个测试类来测试这些形状的`area`方法:
```java
// 测试类 TestShape.java
import org.junit.Test;
import static org.junit.Assert.*;
public class TestShape {
@Test
public void testCircleArea() {
Circle circle = new Circle(5.0);
assertEquals(78.54, circle.area(), 0.001);
}
@Test
public void testRectangleArea() {
Rectangle rectangle = new Rectangle(4.0, 6.0);
assertEquals(24.0, rectangle.area(), 0.001);
}
@Test
public void testTrapezoidArea() {
Trapezoid trapezoid = new Trapezoid(3.0, 7.0, 4.0);
assertEquals(16.0, trapezoid.area(), 0.001);
}
@Test(expected = UnsupportedOperationException.class)
public void testAbstractMethod() {
Shape abstractShape = new Shape(); // 这将抛出异常,因为Shape是抽象的
abstractShape.area();
}
}
```
在这个测试类中,我们使用JUnit框架来进行单元测试,每个测试方法会检查对应形状的`area`计算是否正确。同时,我们还尝试调用`Shape`的抽象方法,这将会导致运行错误,因为我们没有为`Shape`提供实现。
阅读全文
相关推荐
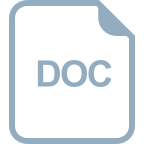
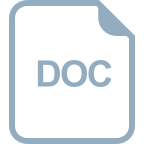
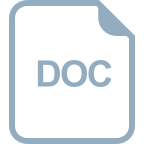














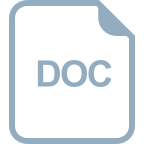
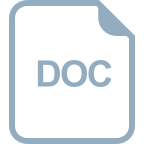