pthread_creat函数
时间: 2024-12-26 11:17:11 浏览: 2
`pthread_create()` 函数是POSIX线程库(pthreads)中的一个关键函数,用于创建新的线程。它在C语言环境中提供了一个接口,允许开发者启动一个新的执行线程,并指定该线程要运行的入口点,即一个函数指针,以及一些附加的数据参数。
函数原型大致如下:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void *), void *arg);
```
参数说明:
- `thread`: 指向新线程标识符的指针,函数返回后将存储新线程的ID。
- `attr`: 可选的线程属性指针,可以用来设置线程的一些特性如优先级等。
- `start_routine`: 要在一个新线程中执行的函数指针,通常是一个void类型的函数,接受一个void*类型的参数(实际上是传给该函数的具体数据),并返回void。
- `arg`: 传递给`start_routine`函数的参数。
使用`pthread_create()` 创建线程后,主线程会继续执行,而新线程会在指定的函数中开始执行。这使得程序能够并发执行多个任务,提高效率。
相关问题
pthread_creat
pthread_create()是一个POSIX线程函数,用于创建一个新线程。它的参数包括一个指向线程标识符的指针,一个指向线程属性的指针,一个指向线程函数的指针,以及一个传递给线程函数的参数。这个函数会在成功创建新线程后返回0,否则返回一个错误码。
要判断两个线程ID是否相等,不能直接使用等号,而是需要使用pthread_equal()函数。这个函数可以判断两个线程ID是否相等,它的参数是两个pthread_t类型的线程ID,返回值为非零表示相等,零表示不相等。
根据实现线程的函数(pthread_creat、pthread_exit、pthread_join),尝试写一个线程编程
以下是一个使用pthread库实现线程的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *thread_function(void *arg) {
int thread_arg = *(int*)arg;
printf("Thread argument: %d\n", thread_arg);
pthread_exit(NULL);
}
int main() {
pthread_t thread;
int thread_arg = 123;
int result = pthread_create(&thread, NULL, thread_function, &thread_arg);
if (result != 0) {
printf("Failed to create thread\n");
exit(EXIT_FAILURE);
}
result = pthread_join(thread, NULL);
if (result != 0) {
printf("Failed to join thread\n");
exit(EXIT_FAILURE);
}
printf("Thread joined\n");
return 0;
}
```
该示例中,我们首先定义了一个线程函数`thread_function`,该函数接受一个整数参数,并在控制台打印该参数的值。然后,在`main`函数中,我们创建了一个线程,并将整数参数传递给线程函数。接下来,我们使用`pthread_join`函数等待线程结束,并检查返回值以确保线程成功加入。最后,我们打印出线程已加入的消息。
阅读全文
相关推荐
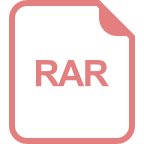
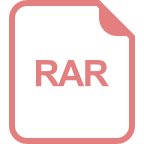














