sprintf(file_name, "File_%d.txt", &i); 为什么有警告
时间: 2024-04-18 22:33:12 浏览: 139
这段代码中出现警告的原因是因为在sprintf函数中,你传递了一个指向变量i的地址,而不是i的值。sprintf函数需要一个整数作为第二个参数,而不是一个指针。
要修复这个警告,你可以将sprintf函数的第二个参数改为i,而不是&i。例如:
sprintf(file_name, "File_%d.txt", i);
相关问题
void file_save(void) { printf("saving"); for (int i = 0; i < 6; i++) { Sleep(200); printf("."); } Sleep(200); file_first = fopen("File_First.txt", "w"); fprintf(file_first, "%5d", file_number); fclose(file_first); current = head; for (int i = 1; i <= file_number; i++) { sprintf(file_name, "File_%d.txt", i); file_current = fopen(file_name, "w"); if (file_current == NULL) { printf("No file existed\n"); system("pause"); system("cls"); } else { current = current->next; rewind(file_current); fprintf(file_current, "%5d%5d%5d%5d%5d%5d%5d%5d\n", current->name_num, current->title_num, current->iden_num, current->pro_num, current->abi_num,current->race_num, current->item_num, current->soc_num); name_current = current->_name; title_current = current->_title; iden_current = current->_iden; pro_current = current->_pro; abi_current = current->_abi; race_current = current->_race; item_current = current->_item; soc_current = current->_soc; for (int i = 0; i < current->name_num; i++) { name_current = name_current->next; fprintf(file_current,"%s\n", name_current->name); } for (int i = 0; i < current->title_num; i++) { title_current = title_current->next; fprintf(file_current,"%s\n", title_current->title); } for (int i = 0; i < current->iden_num; i++) { iden_current = iden_current->next; fprintf(file_current,"%s\n", iden_current->identity); } for (int i = 0; i < current->pro_num; i++) { pro_current = pro_current->next; fprintf(file_current, "%s\n%d\n", pro_current->profession, pro_current->level); } for (int i = 0; i < current->abi_num; i++) { abi_current = abi_current->next; fprintf(file_current, "%s\n%s\n", abi_current->ability, abi_current->explain); } for (int i = 0; i < current->race_num; i++) { race_current = race_current->next; fprintf(file_current, "%s\n", race_current->race); } for (int i = 0; i < current->item_num; i++) { item_current = item_current->next; fprintf(file_current, "%s\n%s\n%d\n", item_current->item, item_current->explain, item_current->number); } for (int i = 0; i < current->soc_num; i++) { soc_current = soc_current->next; fprintf(file_current, "%s\n%s\n%s\n", soc_current->object, soc_current->relationship, soc_current->explain); } fclose(file_current); } } system("files have been saved\n"); system("pause"); system("cls"); } 为什么最后保存的时候保存顺序有很大差别,假设已经有5个文件,为什么不是从File_1保存到File_5
根据你提供的代码,最后保存的顺序是根据链表中节点的顺序来进行保存的。在保存文件之前,你使用了一个循环 `current = current->next` 来遍历链表,这将导致在每次保存文件时,当前节点都会递增到下一个节点。因此,最终保存的文件顺序是根据链表中节点的顺序来决定的。
如果你想按照特定的顺序保存文件,你可以修改代码以满足你的需求。例如,你可以在循环之前定义一个数组来存储要保存的文件顺序,然后根据这个顺序遍历链表并保存文件。
另外,请注意,在循环中使用了相同的变量名 `i`,这可能会导致代码逻辑错误。建议在每个循环中使用不同的变量名来避免混淆。
优化一下代码static void fill_file_chunk(const char *path, int *number, int level) { DIR *dir = NULL; struct dirent *entry = NULL; int i = 0; ++level; if(level > 3) return; dir = opendir(path); if(dir == NULL) return; while((entry = readdir(dir)) != NULL) { if(strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) continue; if(entry->d_type == 4 && level == 1){ if(check_dir_name(entry->d_name) == 0) continue; char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); fill_file_chunk(buffer, number, level); } if(entry->d_type == 4 && level == 2){ if(check_dir_name(entry->d_name) == 0) continue; char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); fill_file_chunk(buffer, number, level); } if(entry->d_type == 8 && level ==3){ if(check_file_name(entry->d_name) == 0) continue; if(*number == max_file_chunk){ file_chunks = (struct FileChunk *)realloc(file_chunks, (max_file_chunk + FILE_CHUNK_INCREAMENT) * sizeof(struct FileChunk)); if(file_chunks == NULL) break; max_file_chunk += FILE_CHUNK_INCREAMENT; } char buffer[MAX_PATH]; sprintf(buffer, "%s/%s", path, entry->d_name); i = *number; strcpy(file_chunks[i].file_name, entry->d_name); sprintf(file_chunks[i].file_path, buffer); file_chunks[i].file_size = get_file_size(buffer); *number = i + 1; } else continue; } closedir(dir); return; }
Here are some suggested optimizations for the given code:
1. Use switch case instead of if-else: In the current code, multiple if-else statements are being used to check for different conditions. This can be optimized by using switch case instead, which can improve readability and maintainability of the code.
2. Use early return to reduce nested if-else: In the current code, multiple if-else statements are nested inside a while loop, which can make the code difficult to read and understand. By using early return, we can reduce the level of nesting and make the code more readable.
3. Avoid redundant code: In the current code, there are multiple instances where the same block of code is being repeated. This can be optimized by refactoring the code and avoiding the duplication of code.
Here is the optimized code:
```
static void fill_file_chunk(const char *path, int *number, int level) {
DIR *dir = opendir(path);
if(dir == NULL) {
return;
}
++level;
if(level > 3) {
closedir(dir);
return;
}
struct dirent *entry;
while((entry = readdir(dir)) != NULL) {
switch(entry->d_type) {
case DT_DIR:
if(level == 1 || level == 2) {
if(check_dir_name(entry->d_name) == 0) {
continue;
}
char buffer[MAX_PATH];
sprintf(buffer, "%s/%s", path, entry->d_name);
fill_file_chunk(buffer, number, level);
}
break;
case DT_REG:
if(level == 3) {
if(check_file_name(entry->d_name) == 0) {
continue;
}
if(*number == max_file_chunk) {
file_chunks = (struct FileChunk *)realloc(file_chunks, (max_file_chunk + FILE_CHUNK_INCREAMENT) * sizeof(struct FileChunk));
if(file_chunks == NULL) {
break;
}
max_file_chunk += FILE_CHUNK_INCREAMENT;
}
char buffer[MAX_PATH];
sprintf(buffer, "%s/%s", path, entry->d_name);
int i = *number;
strcpy(file_chunks[i].file_name, entry->d_name);
sprintf(file_chunks[i].file_path, buffer);
file_chunks[i].file_size = get_file_size(buffer);
*number = i + 1;
}
break;
default:
break;
}
}
closedir(dir);
}
```
This optimized code uses switch case instead of if-else statements, reduces the level of nesting by using early return, and avoids redundant code by refactoring the code.
阅读全文
相关推荐
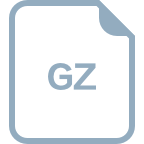
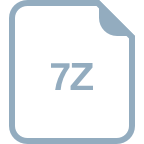
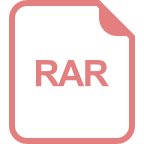














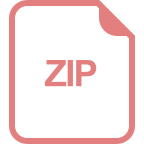