boost 遍历点到点的所有路径
时间: 2023-08-22 14:57:51 浏览: 157
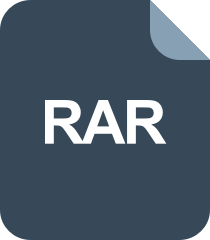
遍历图中两点之间所有路径的算法

在Boost库中,可以使用boost::graph库中的深度优先搜索算法(Depth-First Search,DFS)来遍历点到点之间的所有路径。下面是一个简单的示例代码,演示了如何使用Boost库来实现这个功能:
```cpp
#include <iostream>
#include <boost/graph/adjacency_list.hpp>
#include <boost/graph/depth_first_search.hpp>
// 定义图类型
typedef boost::adjacency_list<boost::listS, boost::vecS, boost::directedS> Graph;
// 定义DFS访问器
struct DFSVisitor : public boost::default_dfs_visitor {
void discover_vertex(Graph::vertex_descriptor v, const Graph& g) const {
std::cout << v << " "; // 输出访问到的顶点
}
};
int main() {
// 创建图
Graph g;
// 添加顶点
Graph::vertex_descriptor v0 = boost::add_vertex(g);
Graph::vertex_descriptor v1 = boost::add_vertex(g);
Graph::vertex_descriptor v2 = boost::add_vertex(g);
Graph::vertex_descriptor v3 = boost::add_vertex(g);
// 添加边
boost::add_edge(v0, v1, g);
boost::add_edge(v1, v2, g);
boost::add_edge(v2, v3, g);
boost::add_edge(v0, v3, g);
// 遍历点到点的所有路径
DFSVisitor visitor;
boost::depth_first_search(g, visitor);
return 0;
}
```
在上述示例代码中,首先我们定义了一个简单的有向图类型Graph,使用`boost::adjacency_list`表示图的结构。然后,我们添加了一些顶点和边,用于构建一个简单的图。
接下来,我们定义了一个DFSVisitor类,继承自`boost::default_dfs_visitor`,重写了其中的`discover_vertex`方法,在该方法中输出访问到的顶点。
最后,在主函数中,我们创建了一个DFSVisitor对象visitor,并使用`boost::depth_first_search`函数来执行深度优先搜索算法。通过这个遍历过程,我们可以输出点到点之间的所有路径。
请注意,这只是一个简单的示例,您可以根据具体需求进行修改和扩展。此外,在实际应用中,您可能还需要处理图中可能存在的环路、路径搜索的终止条件等情况,以获取更加准确和完整的路径信息。
阅读全文
相关推荐
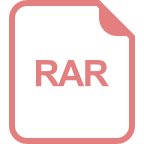
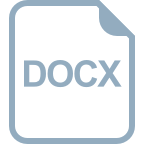
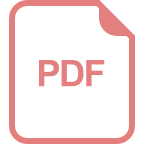
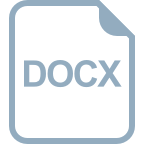
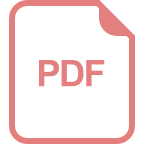
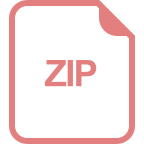
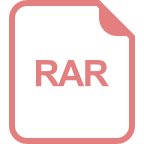
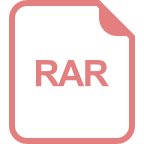
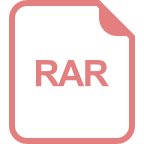
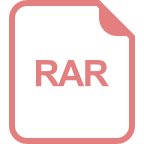





