vue项目canvas绘制文字支持缩放旋转拖拽
时间: 2024-01-28 13:01:41 浏览: 187
Vue项目中,我们可以利用Canvas API来实现文字的绘制,同时支持文字的缩放、旋转和拖拽操作。首先,我们需要在Vue项目中引入Canvas元素,并在对应的组件中进行相关操作。
在绘制文字时,我们可以使用Canvas的fillText()方法来绘制文字,并通过设置字体、颜色、大小等属性来自定义文字的样式。同时,我们可以利用Canvas的transform()方法来实现文字的缩放和旋转操作。通过修改Canvas的transformation matrix,我们可以实现文字的缩放和旋转,从而达到我们想要的效果。
另外,我们还可以通过监听鼠标事件和触摸事件来实现文字的拖拽操作。通过获取用户的鼠标或触摸事件,并结合Canvas中的坐标转换方法,我们可以实现文字的拖拽操作,让用户可以自由地移动文字的位置。
总的来说,在Vue项目中实现Canvas绘制文字并支持缩放、旋转和拖拽,可以通过Canvas API提供的绘制方法和变换方法来实现。通过合理的事件监听和坐标转换,我们可以实现用户交互操作,让用户可以方便地对文字进行操作,从而提升用户体验。
相关问题
帮我实现一个多边形绘制vue组件,具有拖拽、缩放功能
以下是一个简单的多边形绘制 Vue 组件,具有拖拽和缩放功能:
```
<template>
<div class="polygon" :style="polygonStyles">
<div class="handle top-left" @mousedown="startDrag($event, 'top-left')"></div>
<div class="handle top-right" @mousedown="startDrag($event, 'top-right')"></div>
<div class="handle bottom-left" @mousedown="startDrag($event, 'bottom-left')"></div>
<div class="handle bottom-right" @mousedown="startDrag($event, 'bottom-right')"></div>
<canvas ref="canvas" @mousedown="startDraw" @mousemove="draw" @mouseup="endDraw"></canvas>
</div>
</template>
<script>
export default {
data() {
return {
drawing: false,
points: [],
dragHandle: null,
mouseStart: null,
polygonStyles: {
position: 'absolute',
top: '0',
left: '0',
width: '100%',
height: '100%',
cursor: 'crosshair'
}
};
},
mounted() {
this.resizeCanvas();
window.addEventListener('resize', this.resizeCanvas);
},
beforeDestroy() {
window.removeEventListener('resize', this.resizeCanvas);
},
methods: {
resizeCanvas() {
const canvas = this.$refs.canvas;
canvas.width = canvas.offsetWidth;
canvas.height = canvas.offsetHeight;
},
startDraw(event) {
this.drawing = true;
this.mouseStart = this.getMousePosition(event);
this.points = [this.mouseStart];
},
draw(event) {
if (this.drawing) {
const point = this.getMousePosition(event);
const canvas = this.$refs.canvas;
const context = canvas.getContext('2d');
context.clearRect(0, 0, canvas.width, canvas.height);
context.beginPath();
context.moveTo(this.points[0].x, this.points[0].y);
for (let i = 1; i < this.points.length; i++) {
context.lineTo(this.points[i].x, this.points[i].y);
}
context.lineTo(point.x, point.y);
context.stroke();
}
},
endDraw(event) {
this.drawing = false;
this.mouseStart = null;
},
startDrag(event, handle) {
this.dragHandle = handle;
this.mouseStart = this.getMousePosition(event);
},
drag(event) {
if (this.dragHandle) {
const mouseCurrent = this.getMousePosition(event);
const deltaX = mouseCurrent.x - this.mouseStart.x;
const deltaY = mouseCurrent.y - this.mouseStart.y;
const polygon = this.$el;
const polygonRect = polygon.getBoundingClientRect();
const polygonStyles = window.getComputedStyle(polygon);
let newWidth = parseInt(polygonStyles.width) + deltaX;
let newHeight = parseInt(polygonStyles.height) + deltaY;
if (this.dragHandle.includes('left')) {
polygon.style.left = (polygonRect.left + deltaX) + 'px';
newWidth = parseInt(polygonStyles.width) - deltaX;
}
if (this.dragHandle.includes('top')) {
polygon.style.top = (polygonRect.top + deltaY) + 'px';
newHeight = parseInt(polygonStyles.height) - deltaY;
}
polygon.style.width = newWidth + 'px';
polygon.style.height = newHeight + 'px';
this.mouseStart = mouseCurrent;
}
},
endDrag() {
this.dragHandle = null;
this.mouseStart = null;
},
getMousePosition(event) {
const canvas = this.$refs.canvas;
const rect = canvas.getBoundingClientRect();
return {
x: event.clientX - rect.left,
y: event.clientY - rect.top
};
}
}
};
</script>
<style>
.polygon {
position: relative;
display: inline-block;
}
.handle {
position: absolute;
width: 10px;
height: 10px;
background-color: #fff;
border: 1px solid #000;
cursor: move;
}
.top-left {
top: -5px;
left: -5px;
}
.top-right {
top: -5px;
right: -5px;
}
.bottom-left {
bottom: -5px;
left: -5px;
}
.bottom-right {
bottom: -5px;
right: -5px;
}
</style>
```
这个组件包含一个 `canvas` 元素,可以用鼠标绘制多边形。同时,组件还包含四个拖拽手柄,可以用来缩放多边形。当用户按下鼠标时,可以通过 `mousedown` 事件开始绘制多边形或拖拽多边形。当用户移动鼠标时,可以通过 `mousemove` 事件更新多边形或手柄的位置。当用户松开鼠标时,可以通过 `mouseup` 事件结束绘制或拖拽操作。
注意,这个组件只是一个简单的示例,可能不适用于所有情况。如果需要更高级的多边形绘制和缩放功能,可以考虑使用第三方库,如 Konva.js 或 Fabric.js。
vue使用canvas-editor
Vue使用Canvas-Editor是一个基于Vue框架的Canvas编辑器组件,它可以帮助你在网页中创建和编辑Canvas图形。通过Canvas-Editor,你可以轻松地添加、移动、缩放和旋转图形,以及设置图形的样式和属性。
Canvas-Editor提供了一系列的功能和API,使得在Vue项目中使用Canvas变得更加简单和高效。你可以通过Canvas-Editor组件来创建一个Canvas画布,并在画布上添加各种图形元素,如矩形、圆形、线条等。同时,你还可以监听用户的操作事件,如鼠标点击、拖拽等,以实现交互功能。
Canvas-Editor还支持撤销和重做功能,可以方便地回退到之前的编辑状态。此外,它还提供了一些常用的工具和方法,如选择工具、删除工具、复制工具等,以便于用户进行图形编辑操作。
总之,Vue使用Canvas-Editor可以帮助你快速搭建一个功能强大的Canvas编辑器,实现各种图形编辑需求。
阅读全文
相关推荐
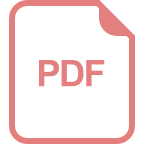
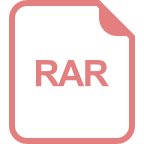
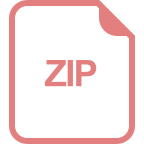
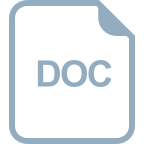
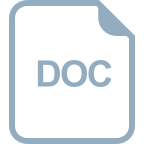




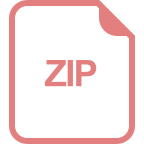
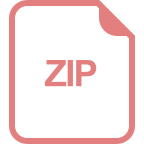
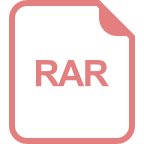
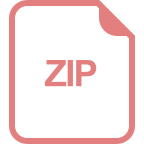

