在python中,我使用priorityqueue存储的元素是一个个对象,priorityqueue如何进行排序
时间: 2023-08-27 10:16:54 浏览: 111
在Python中,如果你使用priority_queue存储的是对象,你可以通过自定义排序函数来实现对priority_queue的排序。你可以使用heapq模块中的方法来实现堆操作,并使用自定义的排序函数来指定对象的优先级。
举个例子,假设你有一个存储Person对象的priority_queue,你可以定义一个比较函数来指定Person对象的排序规则。比较函数应该返回一个负数、零或正数,分别表示第一个对象小于、等于或大于第二个对象。
下面是一个示例代码,演示了如何在Python中使用priority_queue对Person对象进行排序:
```python
import heapq
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __lt__(self, other):
# 自定义对象的排序规则,比较年龄大小
return self.age < other.age
# 创建一个存储Person对象的priority_queue
priority_queue = []
# 添加一些Person对象到priority_queue中
heapq.heappush(priority_queue, Person('Alice', 25))
heapq.heappush(priority_queue, Person('Bob', 30))
heapq.heappush(priority_queue, Person('Charlie', 20))
# 从priority_queue中弹出对象,按照自定义排序规则输出
while priority_queue:
person = heapq.heappop(priority_queue)
print(person.name, person.age)
```
输出结果会按照Person对象的age属性进行排序,从小到大输出。在这个例子中,我们重载了Person类的`__lt__`方法来定义对象的排序规则,使得priority_queue按照年龄的大小进行排序。
希望这个例子能帮助你理解如何在Python中使用priority_queue对对象进行排序。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [STL priority_queue自定义排序实现方法详解](https://blog.csdn.net/weixin_39930557/article/details/111521369)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [[python3] 堆 优先队列(priorityqueue) heapq模块](https://blog.csdn.net/qq_35630119/article/details/119520033)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [Python(一)数据结构和算法的20个练习题问答](https://blog.csdn.net/weixin_39882948/article/details/110994081)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
阅读全文
相关推荐
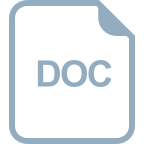
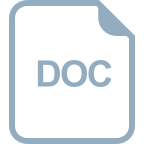
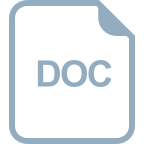

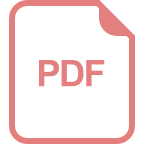
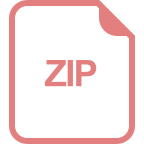
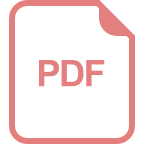
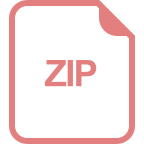
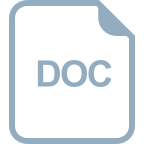
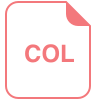
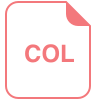
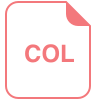
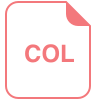
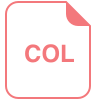
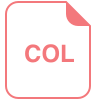
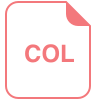
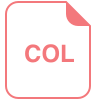

