给定一个字符串s = "hello world",编写一个函数去除字符串中的重复字符,并返回每个字符及其出现次数的字典。
时间: 2024-11-27 08:12:32 浏览: 6
可以使用Python的内置数据结构`collections.Counter`来轻松实现这个功能。首先,将字符串转换成集合以去除重复字符,然后使用`Counter`计算每个字符及其出现次数。以下是相应的函数实现:
```python
from collections import Counter
def remove_duplicates_and_count(s):
unique_chars = set(s) # 使用set去除重复字符
char_dict = Counter(unique_chars) # 使用Counter计算每个字符的频率
return dict(char_dict)
# 示例
s = "hello world"
result = remove_duplicates_and_count(s)
print(result) # 输出:{'l': 3, 'o': 2, 'e': 1, 'h': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1}
```
在这个函数中,`char_dict`是一个字典,键是唯一的字符,值是该字符在原字符串中的出现次数。
相关问题
给定一个字符串 text = "Hello world, this is a Python regex example." ,查找所有单词,并以列表形式返回
为了从给定的字符串 `text = "Hello world, this is a Python regex example."` 中找到所有的单词并以列表形式返回,你可以使用Python的正则表达式库`re`来完成这个任务。下面是一个简单的步骤:
首先,我们需要导入`re`模块,然后定义一个匹配单词的模式(这里是非字母数字字符之间的情况),最后使用`re.findall()`函数来提取所有匹配的单词。
```python
import re
def find_words(text):
pattern = r'\b\w+\b' # 匹配单个单词的正则表达式
words_list = re.findall(pattern, text)
return words_list
text = "Hello world, this is a Python regex example."
word_list = find_words(text)
print(word_list) # 输出:['Hello', 'world,', 'this', 'is', 'a', 'Python', 'regex', 'example.']
编写一个C++函数来计算给定字符串的长度。这个函数需要接受一个字符串作为参数,并返回该字符串的长度。示例输入是一个字符串“Hello,World!”,输出是字符串的长度,即13 给出程序答案
在C++中,你可以使用内置的库函数`std::string::length()`或者`std::string::size()`来获取字符串的长度。下面是一个简单的函数定义示例:
```cpp
#include <iostream>
#include <string>
int stringLength(const std::string& input) {
return input.length(); // 或者 input.size();
}
int main() {
std::string str = "Hello,World!";
int length = stringLength(str);
std::cout << "The length of the string is: " << length << std::endl;
return 0;
}
```
在这个例子中,`stringLength`函数接收一个`const std::string&`类型的引用,表示传递的是一个常量字符串。函数内部直接调用`length()`返回字符串的长度,然后在`main`函数中测试并打印结果。
阅读全文
相关推荐
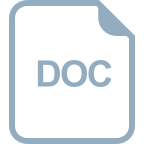
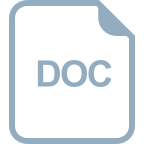
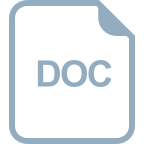













