贝叶斯参数优化逻辑回归Python代码实现
时间: 2024-03-29 13:33:41 浏览: 38
贝叶斯参数优化逻辑回归是一种使用贝叶斯优化算法来寻找逻辑回归模型最佳参数的方法。下面是一个简单的Python代码实现:
```python
import numpy as np
from sklearn.linear_model import LogisticRegression
from skopt import BayesSearchCV
# 准备数据
X = np.array([[1, 2], [3, 4], [5, 6], [7, 8]])
y = np.array([0, 0, 1, 1])
# 定义逻辑回归模型
model = LogisticRegression()
# 定义参数搜索空间
param_space = {
'C': (0.1, 10.0, 'log-uniform'),
'penalty': ['l1', 'l2'],
'fit_intercept': [True, False],
'solver': ['liblinear', 'saga']
}
# 使用贝叶斯参数优化进行参数搜索
opt_model = BayesSearchCV(model, param_space, n_iter=50, cv=3)
opt_model.fit(X, y)
# 输出最佳参数和得分
print("Best parameters found: ", opt_model.best_params_)
print("Best score found: ", opt_model.best_score_)
```
这段代码使用了`sklearn`库中的`LogisticRegression`类作为逻辑回归模型,并使用`skopt`库中的`BayesSearchCV`类进行贝叶斯参数优化。首先,我们准备了输入特征矩阵`X`和目标变量数组`y`。然后,定义了逻辑回归模型`model`和参数搜索空间`param_space`。最后,使用`BayesSearchCV`进行参数搜索,并输出最佳参数和得分。
相关推荐
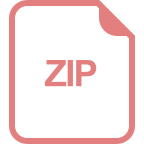
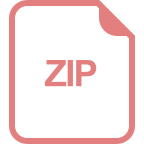
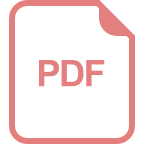














