python批量下载动态网页
时间: 2024-04-09 07:25:59 浏览: 12
在Python中批量下载动态网页的方法有很多种。以下是两种常用的方法:
1. 使用requests库和正则表达式:
```python
import requests
import re
# 发送请求获取网页内容
url = "http://example.com/page"
response = requests.get(url)
html = response.text
# 使用正则表达式提取下载链接
pattern = r'<a href="(.*?)">Download</a>'
download_links = re.findall(pattern, html)
# 遍历下载链接并下载文件
for link in download_links:
file_url = url + link
file_name = link.split("/")[-1]
response = requests.get(file_url)
with open(file_name, "wb") as file:
file.write(response.content)
```
2. 使用BeautifulSoup库:
```python
import requests
from bs4 import BeautifulSoup
# 发送请求获取网页内容
url = "http://example.com/page"
response = requests.get(url)
html = response.text
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(html, "html.parser")
# 找到所有的下载链接
download_links = soup.find_all("a", text="Download")
# 遍历下载链接并下载文件
for link in download_links:
file_url = url + link["href"]
file_name = link["href"].split("/")[-1]
response = requests.get(file_url)
with open(file_name, "wb") as file:
file.write(response.content)
```
这两种方法都可以实现批量下载动态网页中的文件。第一种方法使用了正则表达式来提取下载链接,第二种方法使用了BeautifulSoup库来解析网页内容。你可以根据自己的需求选择其中一种方法来使用。
相关推荐
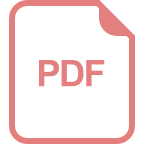














