python批量下载网络图片
时间: 2024-06-21 08:01:41 浏览: 5
在Python中,你可以使用第三方库如`requests`, `beautifulsoup4`(用于解析HTML)和`PIL`(处理图片)来批量下载网络图片。以下是一个简单的步骤说明:
1. 首先,确保安装了这些库,如果没有,可以通过`pip install requests beautifulsoup4 pillow`来安装。
2. 编写一个函数,该函数会根据给定的URL或HTML内容找到所有的图片链接。例如,如果你知道图片在某个HTML页面内的某个类选择器下,可以使用BeautifulSoup来定位。
```python
from bs4 import BeautifulSoup
import requests
def find_image_links(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
img_tags = soup.select('img') # 根据实际HTML结构替换选择器
links = [img['src'] for img in img_tags]
return links
```
3. 对于每个找到的图片链接,创建一个新的请求并保存图片。可以使用`with open()`来保证文件名的唯一性,并且处理可能出现的异常。
```python
def download_images(links, output_folder):
for i, link in enumerate(links, start=1):
file_name = f'image_{i}.jpg' # 这里假设图片都是.jpg格式
try:
response = requests.get(link)
with open(f"{output_folder}/{file_name}", 'wb') as img_file:
img_file.write(response.content)
except Exception as e:
print(f"Failed to download {link}: {e}")
# 使用方法
image_links = find_image_links("http://example.com") # 替换为你想下载图片的网页URL
download_images(image_links, "downloaded_images")
```
4.
相关推荐
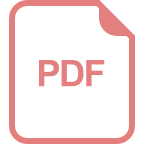
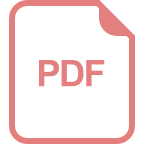














