应用层序遍历生成二叉树并实现遍历(递归算法)
时间: 2024-12-20 19:23:24 浏览: 9
应用层序遍历(也叫前序遍历、中序遍历或后序遍历)生成二叉树是一种常见的数据结构操作。在递归算法中,我们可以这样实现:
1. **前序遍历**(根节点 - 左子树 - 右子树):
```python
def buildTree(preorder, inorder):
if not preorder:
return None
root_val = preorder[0] # 根节点值
root = TreeNode(root_val) # 创建新节点
# 在中序遍历中找到根节点的位置
index = inorder.index(root_val)
# 递归构建左子树和右子树
root.left = buildTree(preorder[1:index], inorder[:index])
root.right = buildTree(preorder[index+1:], inorder[index+1:])
return root
```
2. **中序遍历**(左子树 - 根节点 - 右子树):
```python
def buildTree(preorder, inorder):
...
root.left = buildTree(preorder[:index], inorder[left_idx+1:right_idx])
root.right = buildTree(preorder[right_idx+1:], inorder[right_idx+1:])
...
```
3. **后序遍历**(左子树 - 右子树 - 根节点):
```python
def buildTree(preorder, inorder):
...
root.left = buildTree(preorder[left_idx+1:right_idx], inorder)
root.right = buildTree(preorder[right_idx+1:], inorder)
...
```
在这个过程中,`preorder`数组表示了层序遍历的结果,`inorder`数组用于辅助查找节点位置。通过不断划分待处理的部分,递归地创建子树,并将其连接到根节点上。
阅读全文
相关推荐



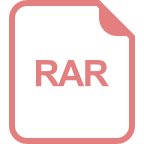
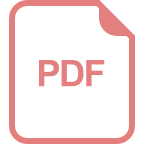
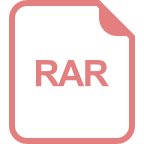
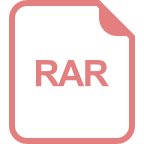
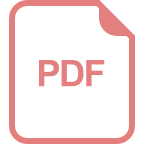
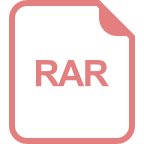
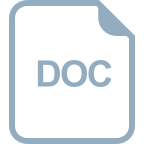
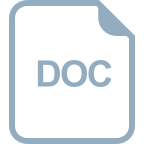
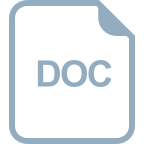
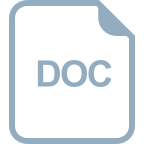
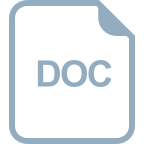
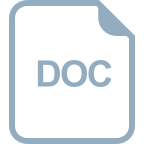
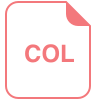

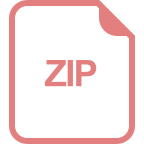